16.后台系统-医院管理
目前把医院、科室和排班都上传到了平台,在管理平台把他们管理起来之后,在管理平台能够直观的查看这些信息
# 展示效果
列表
- 列表中的医院等级是从数据字典中配置获取中文名称
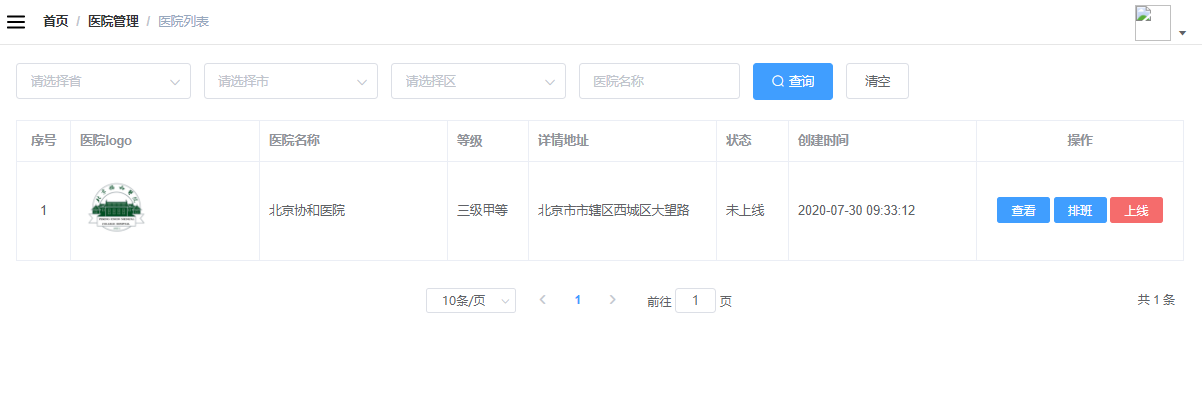
详情
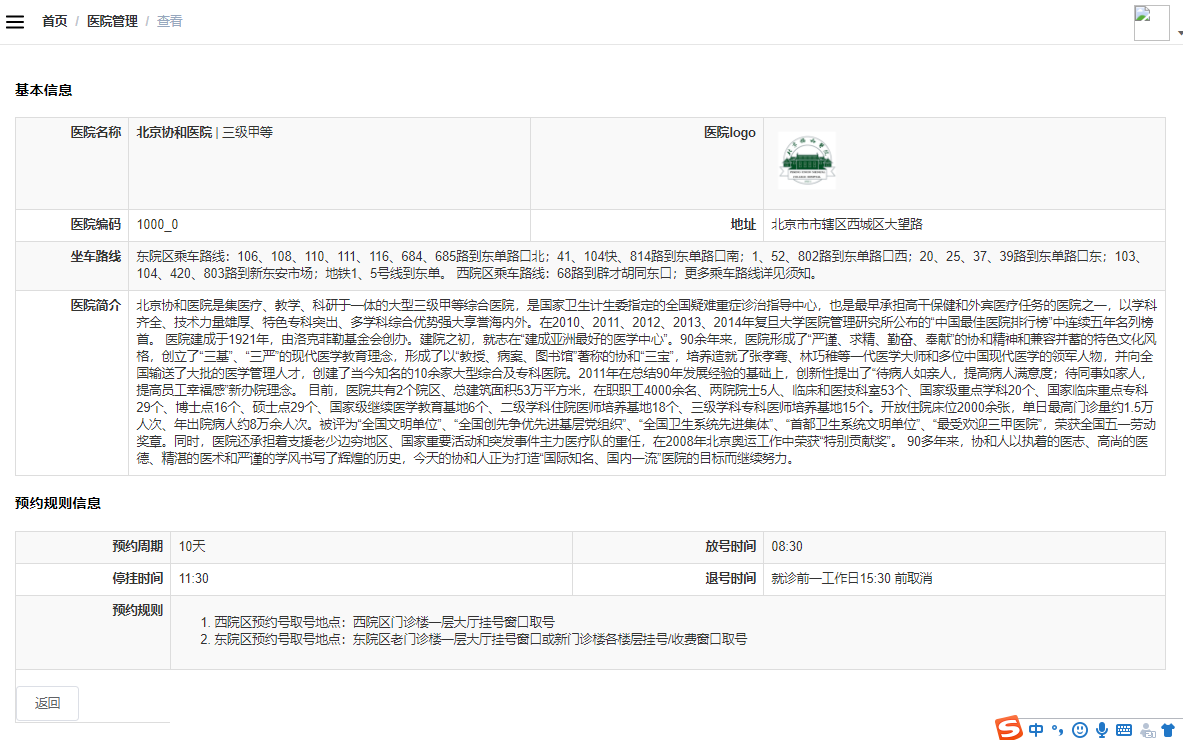
# 医院列表后端实现
# service-hosp中实现列表接口
# model中添加查询vo类
在model
模块添加 HospitalQueryVo
类
package com.stt.yygh.vo.hosp;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import lombok.Data;
import java.io.Serializable;
@Data
@ApiModel(description = "Hospital")
public class HospitalQueryVo implements Serializable {
private static final long serialVersionUID = 1L;
@ApiModelProperty(value = "医院编号")
private String hoscode;
@ApiModelProperty(value = "医院名称")
private String hosname;
@ApiModelProperty(value = "医院类型")
private String hostype;
@ApiModelProperty(value = "省code")
private String provinceCode;
@ApiModelProperty(value = "市code")
private String cityCode;
@ApiModelProperty(value = "区code")
private String districtCode;
@ApiModelProperty(value = "状态")
private Integer status;
}
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
# 添加controller方法
在 service-hosp
模块中创建 HospitalController
类如下
package com.stt.yygh.hosp.controller;
import com.stt.yygh.common.result.Result;
import com.stt.yygh.hosp.service.HospitalService;
import com.stt.yygh.model.hosp.Hospital;
import com.stt.yygh.vo.hosp.HospitalQueryVo;
import io.swagger.annotations.Api;
import io.swagger.annotations.ApiOperation;
import io.swagger.annotations.ApiParam;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.domain.Page;
import org.springframework.web.bind.annotation.*;
@Api(tags = "医院管理接口")
@RestController
@RequestMapping("/admin/hosp/hospital")
@CrossOrigin
public class HospitalController {
@Autowired
private HospitalService service;
@ApiOperation(value = "获取分页列表")
@GetMapping("list/{page}/{limit}")
public Result index(@ApiParam(name = "page", value = "当前页码", required = true)
@PathVariable Integer page,
@ApiParam(name = "limit", value = "每页记录数", required = true)
@PathVariable Integer limit,
@ApiParam(name = "hospitalQueryVo", value = "查询对象", required = false)
HospitalQueryVo hospitalQueryVo) {
Page<Hospital> hospitalPage = service.selectPage(page, limit, hospitalQueryVo);
return Result.ok(hospitalPage);
}
}
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
# 添加service分页接口与实现
在 HospitalService
中添加 selectPage
方法
package com.stt.yygh.hosp.service;
...
public interface HospitalService {
...
Page<Hospital> selectPage(Integer page, Integer limit, HospitalQueryVo hospitalQueryVo);
}
2
3
4
5
6
修改service-hosp
中的 HospitalServiceImpl
类,添加如下方法实现
package com.stt.yygh.hosp.service.impl;
import com.alibaba.fastjson.JSONObject;
import com.stt.yygh.hosp.repository.HospitalRepository;
import com.stt.yygh.hosp.service.HospitalService;
import com.stt.yygh.model.hosp.Hospital;
import com.stt.yygh.vo.hosp.HospitalQueryVo;
import lombok.extern.slf4j.Slf4j;
import org.springframework.beans.BeanUtils;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.domain.*;
import org.springframework.stereotype.Service;
import org.springframework.util.ObjectUtils;
import java.util.Date;
import java.util.Map;
@Slf4j
@Service
public class HospitalServiceImpl implements HospitalService {
@Autowired
private HospitalRepository repository;
...
@Override
public Page<Hospital> selectPage(Integer page, Integer limit, HospitalQueryVo hospitalQueryVo) {
Sort sort = Sort.by(Sort.Direction.DESC, "createTime");
//0为第一页
Pageable pageable = PageRequest.of(page-1, limit, sort);
Hospital hospital = new Hospital();
BeanUtils.copyProperties(hospitalQueryVo, hospital);
//创建匹配器,即如何使用查询条件
ExampleMatcher matcher = ExampleMatcher.matching() //构建对象
.withStringMatcher(ExampleMatcher.StringMatcher.CONTAINING) //改变默认字符串匹配方式:模糊查询
.withIgnoreCase(true); //改变默认大小写忽略方式:忽略大小写
//创建实例
Example<Hospital> example = Example.of(hospital, matcher);
Page<Hospital> pages = repository.findAll(example, pageable);
return pages;
}
}
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
# 测试
使用swagger2页面进行测试 http://localhost:8201/swagger-ui.html 可以传入page 1,limit 10作为参数,查看是否可以正常返回
# service-cmn中提供数据字典查询接口
由于医院等级、省市区地址都是取的数据字典value值,在列表显示医院等级与医院地址时要根据数据字典value值获取数据字典名称
通过数据字典根据上级编码与value值可以获取对应的数据字典名称,如果value值能够保持唯一(不一定唯一),可以直接通过value值获取数据字典名称,目前省市区三级数据我们使用的是国家统计局的数据,数据编码就是数据字典的id与value,所以value能够唯一确定一条数据字典
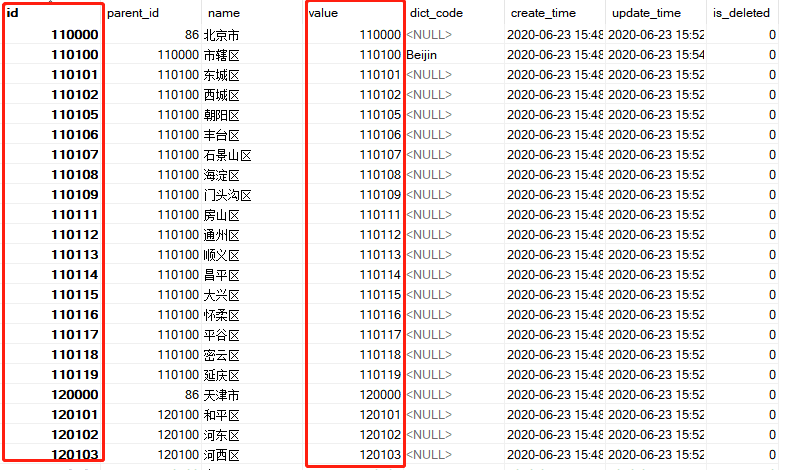
# 添加controller方法
修改 service-cmn
模块中的 DictController
,提供两个api接口,如省市区不需要上级编码,医院等级需要上级编码
- 依据上级编码获以及value值组成的唯一条件,获得该字典的name
- 当value不能唯一定位到字典的name时使用,如医院等级名称
- 依据value获得到字典的name
- 当value可以唯一定位到字典的name时使用,如果省市区名称
package com.stt.yygh.cmn.controller;
...
@Api("数据字典接口")
@RestController
@RequestMapping("/admin/cmn/dict")
@CrossOrigin
public class DictController {
...
@ApiOperation(value = "获取数据字典名称")
@GetMapping(value = "/getName/{parentDictCode}/{value}")
public String getName(@ApiParam(name = "parentDictCode", value = "上级编码", required = true)
@PathVariable("parentDictCode") String parentDictCode,
@ApiParam(name = "value", value = "值", required = true)
@PathVariable("value") String value) {
return dictService.getNameByParentDictCodeAndValue(parentDictCode, value);
}
@ApiOperation(value = "获取数据字典名称")
@ApiImplicitParam(name = "value", value = "值", required = true, dataType = "Long", paramType = "path")
@GetMapping(value = "/getName/{value}")
public String getName(@ApiParam(name = "value", value = "值", required = true)
@PathVariable("value") String value) {
return dictService.getNameByParentDictCodeAndValue("", value);
}
}
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
# 添加service分页接口与实现
在 service-cmn
模块的 DictServiceImpl
添加 getNameByParentDictCodeAndValue
方法声明
package com.stt.yygh.cmn.service;
...
public interface DictService extends IService<Dict> {
...
String getNameByParentDictCodeAndValue(String parentDictCode, String value);
}
2
3
4
5
6
在 service-cmn
模块的 DictServiceImpl
添加实现
- 查询字典的同时,将数据存储在了redis缓存中
package com.stt.yygh.cmn.service.impl;
...
@Service
public class DictServiceImpl extends ServiceImpl<DictMapper, Dict> implements DictService {
...
@Cacheable(value = "dict", keyGenerator = "keyGenerator")
@Override
public String getNameByParentDictCodeAndValue(String parentDictCode, String value) {
//如果value能唯一定位数据字典,parentDictCode可以传空,例如:省市区的value值能够唯一确定
if (StringUtils.isEmpty(parentDictCode)) {
Dict dict = baseMapper.selectOne(new QueryWrapper<Dict>().eq("value", value));
if (Objects.nonNull(dict)) {
return dict.getName();
}
} else {
Dict parentDict = this.getByDictsCode(parentDictCode);
if (Objects.isNull(parentDict)) {
return "";
}
QueryWrapper<Dict> queryWrapper = new QueryWrapper<Dict>()
.eq("parent_id", parentDict.getId())
.eq("value", value);
Dict dict = baseMapper.selectOne(queryWrapper);
if (Objects.nonNull(dict)) {
return dict.getName();
}
}
return "";
}
private Dict getByDictsCode(String parentDictCode) {
Dict dict = baseMapper.selectOne(new QueryWrapper<Dict>().eq("dict_code", parentDictCode));
return dict;
}
}
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
# 测试
在 swagger2中进行测试 http://localhost:8202/swagger-ui.html#!/dict45controller/getNameUsingGET_1
# service-client父模块创建
创建过程同service
父模块,New -> Module->Maven ... -> Finish
删除src等文件夹文件
pom文件修改如下
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<parent>
<artifactId>yygh_parent</artifactId>
<groupId>com.stt.yygh</groupId>
<version>0.0.1</version>
</parent>
<modelVersion>4.0.0</modelVersion>
<artifactId>service-client</artifactId>
<packaging>pom</packaging>
<version>1.0</version>
<properties>
<maven.compiler.source>8</maven.compiler.source>
<maven.compiler.target>8</maven.compiler.target>
</properties>
<dependencies>
<dependency>
<groupId>com.stt.yygh</groupId>
<artifactId>common-util</artifactId>
<version>0.0.1</version>
<scope>provided</scope>
</dependency>
<dependency>
<groupId>com.stt.yygh</groupId>
<artifactId>model</artifactId>
<version>0.0.1</version>
<scope>provided</scope>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
<scope>provided</scope>
</dependency>
<!-- 服务调用feign -->
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-openfeign</artifactId>
<scope>provided</scope>
</dependency>
</dependencies>
</project>
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
# service-cmn-client模块创建(封装feign调用)
创建流程同service-hosp
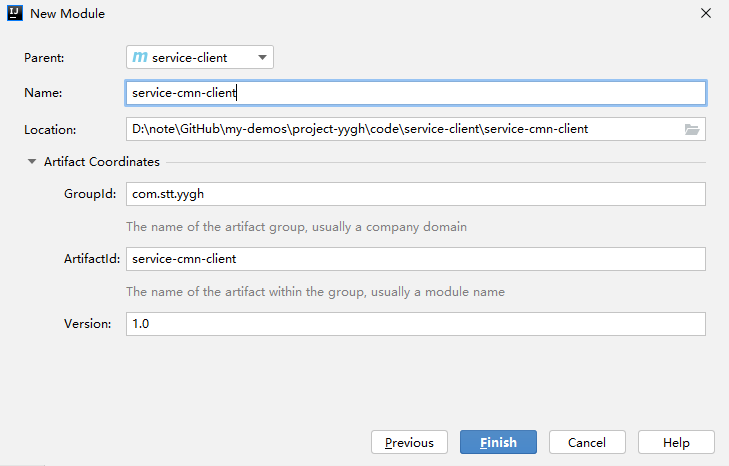
# 修改pom
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<parent>
<artifactId>service-client</artifactId>
<groupId>com.stt.yygh</groupId>
<version>1.0</version>
</parent>
<modelVersion>4.0.0</modelVersion>
<artifactId>service-cmn-client</artifactId>
<packaging>jar</packaging>
<version>1.0</version>
<name>service-cmn-client</name>
<description>service-cmn-client</description>
<properties>
<maven.compiler.source>8</maven.compiler.source>
<maven.compiler.target>8</maven.compiler.target>
</properties>
</project>
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
# 添加feign接口类
创建DictFeignClient
类
注意:使用@PathVariable时必须要有value值
package com.stt.yygh.cmn.client;
import org.springframework.cloud.openfeign.FeignClient;
import org.springframework.stereotype.Component;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
/**
* 数据字典API接口
*/
@FeignClient("service-cmn")
@Component
public interface DictFeignClient {
/**
* 获取数据字典名称
*
* @param parentDictCode
* @param value
* @return
*/
@GetMapping(value = "/admin/cmn/dict/getName/{parentDictCode}/{value}")
String getName(@PathVariable("parentDictCode") String parentDictCode,
@PathVariable("value") String value);
/**
* 获取数据字典名称
*
* @param value
* @return
*/
@GetMapping(value = "/admin/cmn/dict/getName/{value}")
String getName(@PathVariable("value") String value);
}
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
# service-hosp远程调用service-cmn接口
# service模块中引入feign依赖
在service
父模块中添加feign依赖
<!-- 服务调用feign -->
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-openfeign</artifactId>
</dependency>
2
3
4
5
# service-hosp模块添加service-cmn-client依赖
在service-hosp
的pom文件中添加service-cmn-client
依赖
<dependency>
<groupId>com.stt.yygh</groupId>
<artifactId>service-cmn-client</artifactId>
<version>1.0</version>
</dependency>
2
3
4
5
# service-hosp启动类配置
在service-hosp
的启动类中配置开启feign功能
package com.stt.yygh.hosp;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cloud.client.discovery.EnableDiscoveryClient;
import org.springframework.cloud.openfeign.EnableFeignClients;
import org.springframework.context.annotation.ComponentScan;
@SpringBootApplication
@ComponentScan(basePackages = "com.stt")
@EnableDiscoveryClient
@EnableFeignClients(basePackages = "com.stt")
public class ServiceHospApplication {
public static void main(String[] args) {
SpringApplication.run(ServiceHospApplication.class, args);
}
}
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
# model中引入字典枚举类
创建com.stt.yygh.enums
包并添加如下枚举类
package com.stt.yygh.enums;
public enum DictEnum {
HOSTYPE("Hostype", "医院等级"),
CERTIFICATES_TYPE("CertificatesType", "证件类型"),
;
private String dictCode;
private String msg;
DictEnum(String dictCode, String msg) {
this.dictCode = dictCode;
this.msg = msg;
}
public String getDictCode() {
return dictCode;
}
public void setDictCode(String dictCode) {
this.dictCode = dictCode;
}
public String getMsg() {
return msg;
}
public void setMsg(String msg) {
this.msg = msg;
}
}
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
# service-hosp中修改service方法调用service-cmn接口
在HospitalServiceImpl
中修改selectPage逻辑,增加feign接口调用
package com.stt.yygh.hosp.service.impl;
...
@Slf4j
@Service
public class HospitalServiceImpl implements HospitalService {
@Autowired
private DictFeignClient dictFeignClient;
...
@Override
public Page<Hospital> selectPage(Integer page, Integer limit, HospitalQueryVo hospitalQueryVo) {
Sort sort = Sort.by(Sort.Direction.DESC, "createTime");
//0为第一页
Pageable pageable = PageRequest.of(page - 1, limit, sort);
Hospital hospital = new Hospital();
BeanUtils.copyProperties(hospitalQueryVo, hospital);
//创建匹配器,即如何使用查询条件
ExampleMatcher matcher = ExampleMatcher.matching() //构建对象
.withStringMatcher(ExampleMatcher.StringMatcher.CONTAINING) //改变默认字符串匹配方式:模糊查询
.withIgnoreCase(true); //改变默认大小写忽略方式:忽略大小写
//创建实例
Example<Hospital> example = Example.of(hospital, matcher);
Page<Hospital> pages = repository.findAll(example, pageable);
pages.getContent().stream().forEach(h -> this.packHospital(h));
return pages;
}
/**
* 封装数据
*
* @param hospital
* @return
*/
private Hospital packHospital(Hospital hospital) {
// 获取医院等级
String hosptypeStr = dictFeignClient.getName(DictEnum.HOSTYPE.getDictCode(), hospital.getHostype());
// 获取省市区地址
String provinceStr = dictFeignClient.getName(hospital.getProvinceCode());
String cityStr = dictFeignClient.getName(hospital.getCityCode());
String districtStr = dictFeignClient.getName(hospital.getDistrictCode());
// 将查询出的字段放入到param扩展字段中
hospital.getParam().put("hostypeString", hosptypeStr);
hospital.getParam().put("fullAddress", provinceStr + cityStr + districtStr);
return hospital;
}
}
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
修改完成后使用swagger2再测试一下
# service-cmn中添加数据字典显示接口
# 添加controller方法
在DictController
中添加findByDictCode
方法,通过dictCode查询该节点下所有子节点,用于列表页面的下拉选择框的数据展示
package com.stt.yygh.cmn.controller;
...
@Api("数据字典接口")
@RestController
@RequestMapping("/admin/cmn/dict")
@CrossOrigin
public class DictController {
@Autowired
private DictService dictService;
...
@ApiOperation(value = "根据dictCode获取下级节点")
@GetMapping(value = "/findByDictCode/{dictCode}")
public Result<List<Dict>> findByDictCode(@ApiParam(name = "dictCode", value = "节点编码", required = true)
@PathVariable String dictCode) {
List<Dict> list = dictService.findByDictCode(dictCode);
return Result.ok(list);
}
}
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
# 添加service方法
添加接口方法
package com.stt.yygh.cmn.service;
...
public interface DictService extends IService<Dict> {
...
List<Dict> findByDictCode(String dictCode);
}
2
3
4
5
6
添加接口实现
package com.stt.yygh.cmn.service.impl;
...
@Service
public class DictServiceImpl extends ServiceImpl<DictMapper, Dict> implements DictService {
...
@Override
public List<Dict> findByDictCode(String dictCode) {
Dict parentDict = this.getByDictsCode(dictCode);
List<Dict> childData = this.findChildData(parentDict.getId());
return childData;
}
}
2
3
4
5
6
7
8
9
10
11
12
# 医院列表前端实现
# 修改路由
在 src/router/index.js中添加一个路由选项,然后创建views/hosp/list.vue文件
{
path: '/hospSet',
component: Layout,
redirect: '/hospSet/list',
name: '医院设置管理',
meta: { title: '医院设置管理', icon: 'el-icon-s-help' },
children: [
...
{
path: 'hosp/list',
name: '医院列表',
component: () => import('@/views/hosp/list'),
meta: { title: '医院列表', icon: 'table' }
}
]
},
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
# 封装api请求
新建 src/api/hosp.js文件,并填写如下内容
import request from '@/utils/request'
// 医院列表
export function getPageList(current, limit, searchObj) {
return request({
url: `/admin/hosp/hospital/list/${current}/${limit}`,
method: 'get',
params: searchObj // get 请求中的参数使用params,如果data一般用于post请求的requestBody中
})
}
// 查询dictCode查询 数据字典子节点
export function findByDictCode(dictCode) {
return request({
url: `/admin/cmn/dict/findByDictCode/${dictCode}`,
method: 'get'
})
}
// 根据id查询下级数据字典
export function findChildByParentId(id) {
return request({
url: `/admin/cmn/dict/findChildData/${id}`,
method: 'get'
})
}
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
# 添加list.vue组件
创建views/hosp/list.vue文件,并修改
<template>
<div class="app-container">
<el-form :inline="true" class="demo-form-inline">
<el-form-item>
<el-select v-model="searchObj.provinceCode" placeholder="请选择省" @change="provinceChanged">
<el-option v-for="item in provinceList" :key="item.id" :label="item.name" :value="item.id"/>
</el-select>
</el-form-item>
<el-form-item>
<el-select v-model="searchObj.cityCode" placeholder="请选择市" @change="cityChanged">
<el-option v-for="item in cityList" :key="item.id" :label="item.name" :value="item.id"/>
</el-select>
</el-form-item>
<el-form-item>
<el-input v-model="searchObj.hosname" placeholder="医院名称"/>
</el-form-item>
<el-button type="primary" icon="el-icon-search" @click="fetchData()">查询</el-button>
<el-button type="default" @click="resetData()">清空</el-button>
</el-form>
<el-table v-loading="listLoading" :data="list" border fit highlight-current-row>
<el-table-column label="序号" width="60" align="center">
<template slot-scope="scope">{{ (page - 1) * limit + scope.$index + 1 }}</template>
</el-table-column>
<el-table-column label="医院logo">
<template slot-scope="scope">
<img :src="'data:image/jpeg;base64,'+scope.row.logoData" width="80">
</template>
</el-table-column>
<el-table-column prop="hosname" label="医院名称"/>
<el-table-column prop="param.hostypeString" label="等级" width="90"/>
<el-table-column prop="param.fullAddress" label="详情地址"/>
<el-table-column label="状态" width="80">
<template slot-scope="scope">{{ scope.row.status === 0 ? '未上线' : '已上线' }}</template>
</el-table-column>
<el-table-column prop="createTime" label="创建时间"/>
<el-table-column label="操作" width="230" align="center"/>
</el-table>
<!-- 分页组件 -->
<el-pagination :current-page="page" :total="total" :page-size="limit"
:page-sizes="[5, 10, 20, 30, 40, 50, 100]"
style="padding: 30px 0; text-align: center;"
layout="sizes, prev, pager, next, jumper, ->, total, slot"
@current-change="fetchData" @size-change="changeSize"/>
</div>
</template>
<script>
import { findByDictCode, findChildByParentId, getPageList } from '@/api/hosp'
export default {
data() {
return {
listLoading: true, // 数据是否正在加载
list: null, // banner列表
total: 0, // 数据库中的总记录数
page: 1, // 默认页码
limit: 10, // 每页记录数
searchObj: {}, // 查询表单对象
provinceList: [],
cityList: [],
districtList: []
}
},
// 生命周期函数:内存准备完毕,页面尚未渲染
created() {
console.log('list created......')
this.fetchData()
findByDictCode('Province').then(res => {
this.provinceList = res.data
})
},
methods: {
fetchData(page = 1) {
console.log('翻页...', page)
this.page = page
this.listLoading = true
getPageList(this.page, this.limit, this.searchObj).then(
response => {
this.list = response.data.content
this.total = response.data.totalElements
// 数据加载并绑定成功
this.listLoading = false
}
)
},
changeSize(size) { // 当页码发生改变的时候
this.limit = size
this.fetchData(1)
},
resetData() {
console.log('重置查询表单')
this.searchObj = {}
this.fetchData()
},
provinceChanged() {
this.cityList = []
this.districtList = []
this.searchObj.cityCode = null
this.searchObj.districtCode = null
findChildByParentId(this.searchObj.provinceCode).then(res => {
this.cityList = res.data
})
},
cityChanged() {
// 待完善
}
}
}
</script>
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
# 更新医院上线状态后端实现
# 添加controller
在service-hosp
的HospitalController
中添加接口方法
package com.stt.yygh.hosp.controller;
...
@Api(tags = "医院管理接口")
@RestController
@RequestMapping("/admin/hosp/hospital")
@CrossOrigin
public class HospitalController {
...
@ApiOperation(value = "更新上线状态")
@GetMapping("updateStatus/{id}/{status}")
public Result lock(@ApiParam(name = "id", value = "医院id", required = true)
@PathVariable("id") String id,
@ApiParam(name = "status", value = "状态(0:未上线 1:已上线)", required = true)
@PathVariable("status") Integer status) {
service.updateStatus(id, status);
return Result.ok();
}
}
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
# 添加service
在service-hosp
的HospitalService
添加接口
package com.stt.yygh.hosp.service;
...
public interface HospitalService {
...
void updateStatus(String id, Integer status);
}
2
3
4
5
6
在service-hosp
的HospitalService
添加接口实现
package com.stt.yygh.hosp.service.impl;
...
@Slf4j
@Service
public class HospitalServiceImpl implements HospitalService {
...
@Override
public void updateStatus(String id, Integer status) {
if(status.intValue() == 0 || status.intValue() == 1) {
Hospital hospital = repository.findById(id).get();
hospital.setStatus(status);
hospital.setUpdateTime(new Date());
repository.save(hospital);
}
}
}
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
# 更新医院上线状态前端实现
# 封装api请求
修改 src/api/hosp.js文件,添加如下内容
export function updateStatus(id, status) {
return request({
url: `/admin/hosp/hospital/updateStatus/${id}/${status}`,
method: 'get'
})
}
2
3
4
5
6
# 添加vue组件
修改views/hosp/list.vue文件,添加如下内容
<template>
<div class="app-container">
...
<el-table v-loading="listLoading" :data="list" border fit highlight-current-row>
...
<el-table-column label="操作" width="230" align="center">
<template slot-scope="scope">
<el-button v-if="scope.row.status == 1" type="primary" size="mini"
@click="updateStatus(scope.row.id, 0)">下线
</el-button>
<el-button v-if="scope.row.status == 0" type="danger" size="mini"
@click="updateStatus(scope.row.id, 1)">上线
</el-button>
</template>
</el-table-column>
</el-table>
...
</div>
</template>
<script>
import { findByDictCode, findChildByParentId, getPageList, updateStatus } from '@/api/hosp'
export default {
data() {
return {
...
}
},
...
methods: {
...
updateStatus(id, status) {
updateStatus(id, status)
.then(response => {
this.fetchData(this.page)
})
}
}
}
</script>
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
# 医院详情后端实现
# 添加controller
在service-hosp
的HospitalController
中添加接口方法
package com.stt.yygh.hosp.controller;
...
@Api(tags = "医院管理接口")
@RestController
@RequestMapping("/admin/hosp/hospital")
@CrossOrigin
public class HospitalController {
...
@ApiOperation(value = "获取医院详情")
@GetMapping("show/{id}")
public Result show(@ApiParam(name = "id", value = "医院id", required = true)
@PathVariable String id) {
Map<String, Object> detail = service.show(id);
return Result.ok(detail);
}
}
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
# 添加service
在service-hosp
的HospitalService
添加接口
package com.stt.yygh.hosp.service;
...
public interface HospitalService {
...
/**
* 医院详情
* @param id
* @return
*/
Map<String, Object> show(String id);
}
2
3
4
5
6
7
8
9
10
11
在service-hosp
的HospitalService
添加接口实现
package com.stt.yygh.hosp.service.impl;
...
@Slf4j
@Service
public class HospitalServiceImpl implements HospitalService {
@Autowired
private HospitalRepository repository;
...
@Override
public Map<String, Object> show(String id) {
Map<String, Object> result = new HashMap<>();
Hospital hospital = this.packHospital(repository.findById(id).get());
result.put("hospital", hospital);
//单独处理更直观
result.put("bookingRule", hospital.getBookingRule());
//不需要重复返回
hospital.setBookingRule(null);
// 返回值最好使用一个VO,这样里面的字段都很清晰,虽然返回Map扩展性很高,但是不好维护
return result;
}
}
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
# 医院详情前端实现
# 添加隐藏路由
{
path: '/hospSet',
component: Layout,
redirect: '/hospSet/list',
name: '医院设置管理',
meta: { title: '医院设置管理', icon: 'el-icon-s-help' },
children: [
...
{
path: 'hosp/list',
name: '医院列表',
component: () => import('@/views/hosp/list'),
meta: { title: '医院列表', icon: 'table' }
},
{
path: 'hosp/show/:id',
name: '查看',
component: () => import('@/views/hosp/show'),
meta: { title: '查看', noCache: true },
hidden: true
}
]
},
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
# 封装api请求
修改 src/api/hosp.js文件,添加如下内容
// 查看医院详情
export function getHospById(id) {
return request({
url: `/admin/hosp/hospital/show/${id}`,
method: 'get'
})
}
2
3
4
5
6
7
# 修改list.vue组件
修改views/hosp/list.vue文件,添加查看按键
<template>
<div class="app-container">
...
<el-table v-loading="listLoading" :data="list" border fit highlight-current-row>
...
<el-table-column label="操作" width="230" align="center">
<template slot-scope="scope">
<el-button v-if="scope.row.status == 1" type="primary" size="mini"
@click="updateStatus(scope.row.id, 0)">下线
</el-button>
<el-button v-if="scope.row.status == 0" type="danger" size="mini"
@click="updateStatus(scope.row.id, 1)">上线
</el-button>
<router-link :to="'/hospSet/hosp/show/'+scope.row.id">
<el-button type="primary" size="mini">查看</el-button>
</router-link>
</template>
</el-table-column>
</el-table>
...
</div>
</template>
...
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
# 创建show.vue组件
创建views/hosp/show.vue文件,并修改
<template>
<div class="app-container">
<h4>基本信息</h4>
<table class="table table-striped table-condenseda table-bordered" width="100%">
<tbody>
<tr>
<th width="15%">医院名称</th>
<td width="35%"><b style="font-size: 14px">{{ hospital.hosname }}</b> | {{ hospital.param.hostypeString }}</td>
<th width="15%">医院logo</th>
<td width="35%">
<img :src="'data:image/jpeg;base64,'+hospital.logoData" width="80">
</td>
</tr>
<tr>
<th>医院编码</th>
<td>{{ hospital.hoscode }}</td>
<th>地址</th>
<td>{{ hospital.param.fullAddress }}</td>
</tr>
<tr>
<th>坐车路线</th>
<td colspan="3">{{ hospital.route }}</td>
</tr>
<tr>
<th>医院简介</th>
<td colspan="3">{{ hospital.intro }}</td>
</tr>
</tbody>
</table>
<h4>预约规则信息</h4>
<table class="table table-striped table-condenseda table-bordered" width="100%">
<tbody>
<tr>
<th width="15%">预约周期</th>
<td width="35%">{{ bookingRule.cycle }}天</td>
<th width="15%">放号时间</th>
<td width="35%">{{ bookingRule.releaseTime }}</td>
</tr>
<tr>
<th>停挂时间</th>
<td>{{ bookingRule.stopTime }}</td>
<th>退号时间</th>
<td>{{ bookingRule.quitDay == -1 ? '就诊前一工作日' : '就诊当日' }}{{ bookingRule.quitTime }} 前取消</td>
</tr>
<tr>
<th>预约规则</th>
<td colspan="3">
<ol>
<li v-for="item in bookingRule.rule" :key="item">{{ item }}</li>
</ol>
</td>
</tr>
<br/>
<el-row>
<el-button @click="back">返回</el-button>
</el-row>
</tbody>
</table>
</div>
</template>
<script>
import { getHospById } from '@/api/hosp'
export default {
data() {
return {
hospital: null, // 医院信息
bookingRule: null // 预约信息
}
},
created() {
// 获取路由id
const id = this.$route.params.id
// 调用方法,根据id查询医院详情
this.fetchHospDetail(id)
},
methods: {
// 根据id查询医院详情
fetchHospDetail(id) {
getHospById(id)
.then(res => {
this.hospital = res.data.hospital
this.bookingRule = res.data.bookingRule
})
},
back() { // 返回医院列表
this.$router.push({ path: '/hospSet/hosp/list' })
}
}
}
</script>
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
# 添加样式
改样式文件是控制详情展示的css布局文件
- 在yygh-admin/src/styles目录下创建show.css
- 在src/main.js文件添加引用
show.css内容如下
.app-container{
background: #fff;
position: absolute;
width:100%;
height: 100%;
overflow: auto;
}
table {
border-spacing: 0;
border-collapse: collapse;
}
.table-bordered {
border: 1px solid #ddd;
}
.table-striped>tbody>tr:nth-child(odd)>td, .table-striped>tbody>tr:nth-child(odd)>th {
background-color: #f9f9f9;
}
.table>thead>tr>th, .table>tbody>tr>th, .table>tfoot>tr>th,
.table>thead>tr>td, .table>tbody>tr>td, .table>tfoot>tr>td {
font-size: 14px;
color: #333;
padding: 8px;
line-height: 1.42857143;
vertical-align: top;
border-top: 1px solid #ddd;
}
.table>thead>tr>th, .table>tbody>tr>th, .table>tfoot>tr>th{
text-align: right;
width: 120px;
}
.table-bordered>thead>tr>th, .table-bordered>tbody>tr>th, .table-bordered>tfoot>tr>th, .table-bordered>thead>tr>td, .table-bordered>tbody>tr>td, .table-bordered>tfoot>tr>td {
border: 1px solid #ddd;
}
.active_content{
padding: 0 20px ;
border-radius: 4px;
border: 1px solid #ebeef5;
background-color: #fff;
overflow: hidden;
color: #303133;
transition: .3s;
box-shadow: 0 2px 12px 0 rgba(0,0,0,.1);
}
.active_content h4{
/*line-height: 0;*/
}
.active_content span{
font-size: 12px;
color: #999;
}
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
在main.js中引入 show.css
import Vue from 'vue'
...
import '@/styles/index.scss' // global css
import '@/styles/show.css'
...
2
3
4
5