32.前台系统-订单管理
# 需求分析
订单列表
之前实现了预约下单功能,创建完成订单后,要在前台系统用户可以进行管理
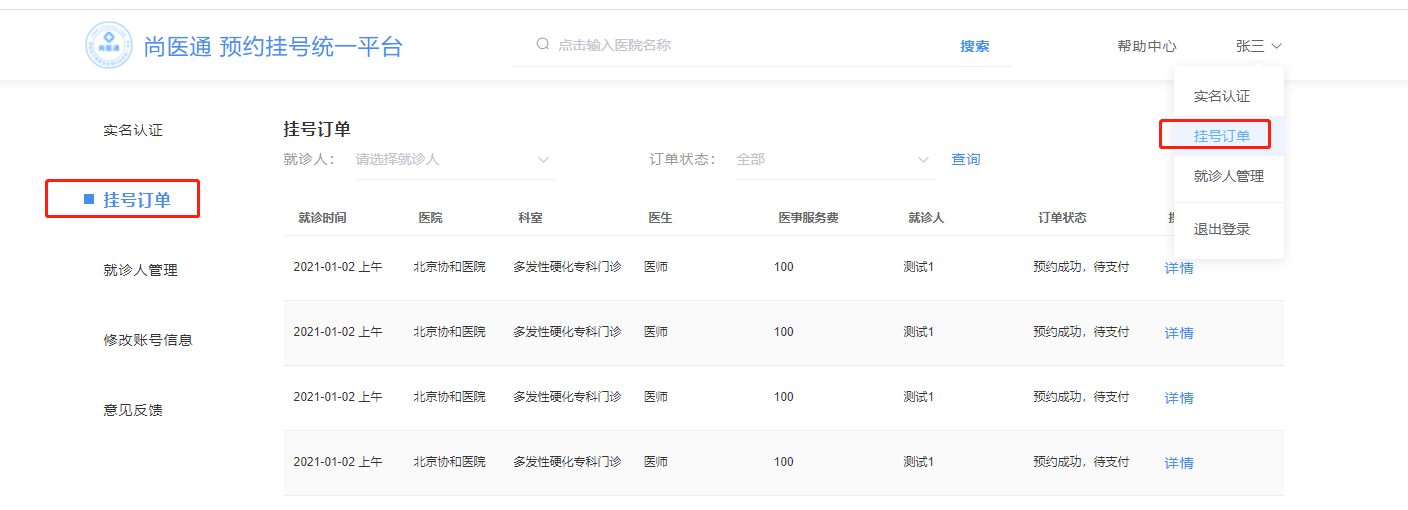
订单详情
点击详情后,可以看到订单详情页面
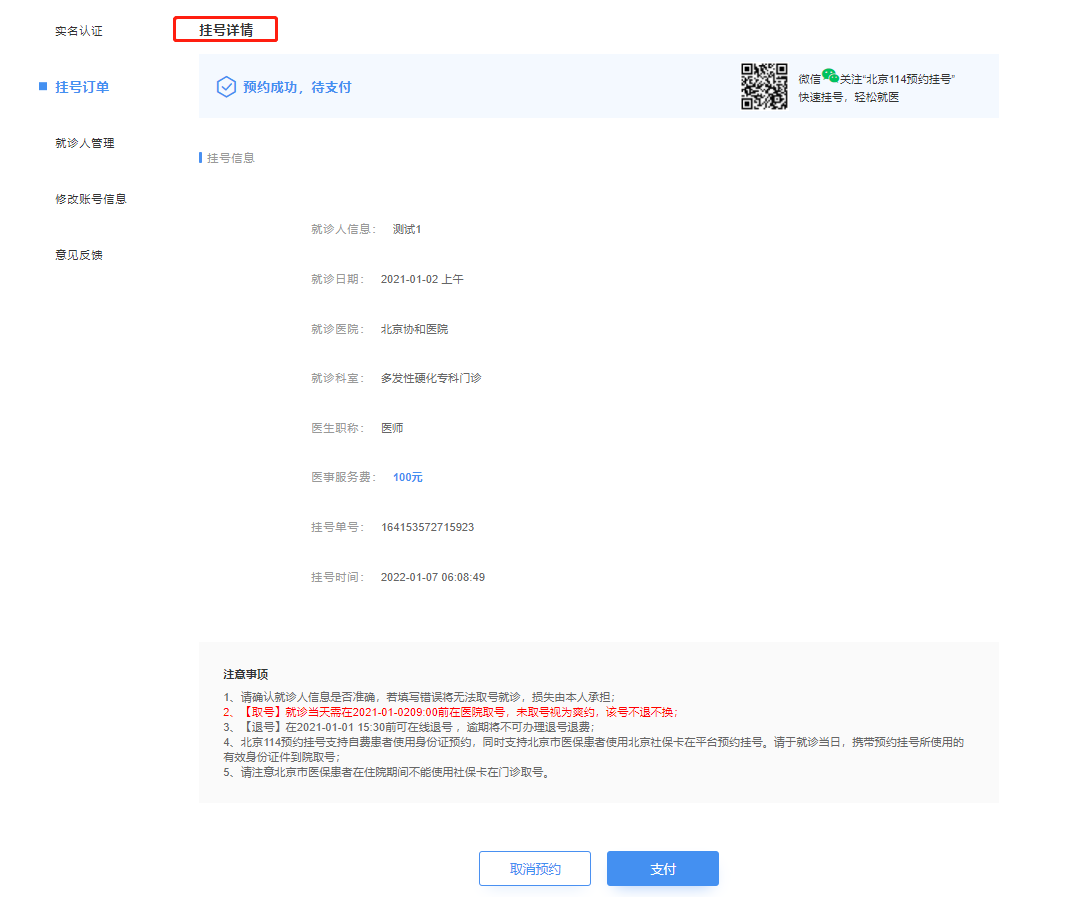
# 订单列表 service-order
# 添加查询实体 model
在model
中添加实体类 com.stt.yygh.vo.order.OrderQueryVo
package com.stt.yygh.vo.order;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import lombok.Data;
@Data
@ApiModel(description = "Order")
public class OrderQueryVo {
@ApiModelProperty(value = "会员id")
private Long userId;
@ApiModelProperty(value = "订单交易号")
private String outTradeNo;
@ApiModelProperty(value = "就诊人id")
private Long patientId;
@ApiModelProperty(value = "就诊人")
private String patientName;
@ApiModelProperty(value = "医院名称")
private String keyword;
@ApiModelProperty(value = "订单状态")
private String orderStatus;
@ApiModelProperty(value = "安排日期")
private String reserveDate;
@ApiModelProperty(value = "创建时间")
private String createTimeBegin;
private String createTimeEnd;
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
# 添加service方法与实现
在 service-order
模块中的OrderService
添加分页查询方法
package com.stt.yygh.order.service;
import com.baomidou.mybatisplus.extension.plugins.pagination.Page;
import com.baomidou.mybatisplus.extension.service.IService;
import com.stt.yygh.model.order.OrderInfo;
import com.stt.yygh.vo.order.OrderQueryVo;
public interface OrderService extends IService<OrderInfo> {
...
// 分页列表
Page<OrderInfo> selectPage(Page<OrderInfo> pageParam, OrderQueryVo orderQueryVo);
}
1
2
3
4
5
6
7
8
9
10
11
12
2
3
4
5
6
7
8
9
10
11
12
对应的实现
package com.stt.yygh.order.service.impl;
import com.alibaba.fastjson.JSONObject;
import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper;
import com.baomidou.mybatisplus.extension.plugins.pagination.Page;
import com.baomidou.mybatisplus.extension.service.impl.ServiceImpl;
import com.stt.yygh.common.exception.YyghException;
import com.stt.yygh.common.helper.HttpRequestHelper;
import com.stt.yygh.common.result.ResultCodeEnum;
import com.stt.yygh.enums.OrderStatusEnum;
import com.stt.yygh.hosp.client.HospitalFeignClient;
import com.stt.yygh.model.order.OrderInfo;
import com.stt.yygh.model.user.Patient;
import com.stt.yygh.order.mapper.OrderInfoMapper;
import com.stt.yygh.order.service.OrderService;
import com.stt.yygh.rabbitmq.MqConst;
import com.stt.yygh.rabbitmq.RabbitService;
import com.stt.yygh.user.client.PatientFeignClient;
import com.stt.yygh.vo.order.OrderMqVo;
import com.stt.yygh.vo.hosp.ScheduleOrderVo;
import com.stt.yygh.vo.hosp.SignInfoVo;
import com.stt.yygh.vo.msm.MsmVo;
import com.stt.yygh.vo.order.OrderQueryVo;
import org.joda.time.DateTime;
import org.springframework.beans.BeanUtils;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import org.springframework.transaction.annotation.Transactional;
import org.springframework.util.StringUtils;
import java.util.HashMap;
import java.util.Map;
import java.util.Objects;
import java.util.Random;
@Service
public class OrderServiceImpl extends ServiceImpl<OrderInfoMapper, OrderInfo> implements OrderService {
...
@Override
public Page<OrderInfo> selectPage(Page<OrderInfo> pageParam, OrderQueryVo orderQueryVo) {
String name = orderQueryVo.getKeyword(); //医院名称
Long patientId = orderQueryVo.getPatientId(); //就诊人名称
String orderStatus = orderQueryVo.getOrderStatus(); //订单状态
String reserveDate = orderQueryVo.getReserveDate(); //安排时间
String createTimeBegin = orderQueryVo.getCreateTimeBegin();
String createTimeEnd = orderQueryVo.getCreateTimeEnd();
//对条件值进行非空判断
QueryWrapper<OrderInfo> wrapper = new QueryWrapper<>();
if (!StringUtils.isEmpty(name)) wrapper.like("hosname", name);
if (!Objects.isNull(patientId)) wrapper.eq("patient_id", patientId);
if (!StringUtils.isEmpty(orderStatus)) wrapper.eq("order_status", orderStatus);
if (!StringUtils.isEmpty(reserveDate)) wrapper.ge("reserve_date", reserveDate);
if (!StringUtils.isEmpty(createTimeBegin)) wrapper.ge("create_time", createTimeBegin);
if (!StringUtils.isEmpty(createTimeEnd)) wrapper.le("create_time", createTimeEnd);
Page<OrderInfo> pages = baseMapper.selectPage(pageParam, wrapper);
pages.getRecords().forEach(this::packOrderInfo);
return pages;
}
private OrderInfo packOrderInfo(OrderInfo orderInfo) {
orderInfo.getParam().put("orderStatusString", OrderStatusEnum.getStatusNameByStatus(orderInfo.getOrderStatus()));
return orderInfo;
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
# 添加controller
订单状态封装到枚举中的,页面搜索需要一个下拉列表展示,通过接口返回页面
package com.stt.yygh.order.controller;
import com.baomidou.mybatisplus.extension.plugins.pagination.Page;
import com.stt.yygh.common.result.Result;
import com.stt.yygh.common.utils.AuthContextHolder;
import com.stt.yygh.enums.OrderStatusEnum;
import com.stt.yygh.model.order.OrderInfo;
import com.stt.yygh.order.service.OrderService;
import com.stt.yygh.vo.order.OrderQueryVo;
import io.swagger.annotations.Api;
import io.swagger.annotations.ApiOperation;
import io.swagger.annotations.ApiParam;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.*;
import javax.servlet.http.HttpServletRequest;
@Api(tags = "订单接口")
@RestController
@RequestMapping("/api/order/orderInfo")
public class OrderApiController {
@Autowired
private OrderService service;
...
//订单列表(条件查询带分页)
@GetMapping("auth/{page}/{limit}")
public Result list(@PathVariable Long page,
@PathVariable Long limit,
OrderQueryVo orderQueryVo, HttpServletRequest request) {
//设置当前用户id
orderQueryVo.setUserId(AuthContextHolder.getUserId(request));
Page<OrderInfo> pageModel = service.selectPage(new Page<>(page,limit),orderQueryVo);
return Result.ok(pageModel);
}
@ApiOperation(value = "获取订单状态")
@GetMapping("auth/getStatusList")
public Result getStatusList() {
return Result.ok(OrderStatusEnum.getStatusList());
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
# 订单列表 yygh-site
# 封装api
在/api/order/orderInfo.js添加方法
import request from '@/utils/request'
const api_name = `/api/order/orderInfo`
...
//订单列表
export function getPageList(page, limit, searchObj) {
return request({
url: `${api_name}/auth/${page}/${limit}`,
method: `get`,
params: searchObj
})
}
//订单状态
export function getStatusList() {
return request({
url: `${api_name}/auth/getStatusList`,
method: 'get'
})
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
# 创建页面组件
创建/pages/order/index.vue组件
<template>
<!-- header -->
<div class="nav-container page-component">
<!--左侧导航 #start -->
<div class="nav left-nav">
<div class="nav-item ">
<span class="v-link clickable dark" onclick="javascript:window.location='/user'">实名认证</span>
</div>
<div class="nav-item selected">
<span class="v-link selected dark" onclick="javascript:window.location='/order'">挂号订单</span>
</div>
<div class="nav-item ">
<span class="v-link clickable dark" onclick="javascript:window.location='/patient'">就诊人管理</span>
</div>
<div class="nav-item "><span class="v-link clickable dark">修改账号信息</span></div>
<div class="nav-item "><span class="v-link clickable dark">意见反馈</span></div>
</div>
<!-- 左侧导航 #end -->
<!-- 右侧内容 #start -->
<div class="page-container">
<div class="personal-order">
<div class="title"> 挂号订单</div>
<el-form :inline="true">
<el-form-item label="就诊人:">
<el-select v-model="searchObj.patientId" placeholder="请选择就诊人" class="v-select patient-select">
<el-option v-for="item in patientList" :key="item.id"
:label="item.name + '【' + item.certificatesNo + '】'" :value="item.id"/>
</el-select>
</el-form-item>
<el-form-item label="订单状态:" style="margin-left: 80px">
<el-select v-model="searchObj.orderStatus" placeholder="全部" class="v-select patient-select" style="width: 200px;">
<el-option v-for="item in statusList" :key="item.status" :label="item.comment" :value="item.status"/>
</el-select>
</el-form-item>
<el-form-item>
<el-button type="text" class="search-button v-link highlight clickable selected" @click="fetchData()">
查询
</el-button>
</el-form-item>
</el-form>
<div class="table-wrapper table">
<el-table :data="list" stripe style="width: 100%">
<el-table-column label="就诊时间" width="120">
<template slot-scope="scope">
{{ scope.row.reserveDate }} {{ scope.row.reserveTime === 0 ? '上午' : '下午' }}
</template>
</el-table-column>
<el-table-column prop="hosname" label="医院" width="100"/>
<el-table-column prop="depname" label="科室" />
<el-table-column prop="title" label="医生" />
<el-table-column prop="amount" label="医事服务费" />
<el-table-column prop="patientName" label="就诊人" />
<el-table-column prop="param.orderStatusString" label="订单状态" />
<el-table-column label="操作">
<template slot-scope="scope">
<el-button type="text" class="v-link highlight clickable selected" @click="show(scope.row.id)">详情</el-button>
</template>
</el-table-column>
</el-table>
</div>
<!-- 分页 -->
<el-pagination class="pagination" layout="prev, pager, next"
:current-page="page" :total="total" :page-size="limit" @current-change="fetchData"/>
</div>
</div>
<!-- 右侧内容 #end -->
</div>
<!-- footer -->
</template>
<script>
import '~/assets/css/hospital_personal.css'
import '~/assets/css/hospital.css'
import { getPageList, getStatusList } from '@/api/order/orderInfo'
import { findList } from '@/api/user/patient'
export default {
data() {
return {
list: [], // banner列表
total: 0, // 数据库中的总记录数
page: 1, // 默认页码
limit: 10, // 每页记录数
searchObj: {}, // 查询表单对象
patientList: [],
statusList: []
}
},
created() {
this.orderId = this.$route.query.orderId
this.fetchData()
this.findPatientList()
this.getStatusList()
},
methods: {
fetchData(page = 1) {
this.page = page
getPageList(this.page, this.limit, this.searchObj).then(res => {
console.log(res.data);
this.list = res.data.records
this.total = res.data.total
})
},
findPatientList() {
findList().then(res => {
this.patientList = res.data
})
},
getStatusList() {
getStatusList().then(res => {
this.statusList = res.data
})
},
changeSize(size) {
this.limit = size
this.fetchData(1)
},
show(id) {
window.location.href = '/order/show?orderId=' + id
}
}
}
</script>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
# 订单详情 service-order
# 添加service方法与实现
添加接口
package com.stt.yygh.order.service;
import com.baomidou.mybatisplus.extension.plugins.pagination.Page;
import com.baomidou.mybatisplus.extension.service.IService;
import com.stt.yygh.model.order.OrderInfo;
import com.stt.yygh.vo.order.OrderQueryVo;
public interface OrderService extends IService<OrderInfo> {
...
// 获取订单详情
OrderInfo getOrder(Long id);
}
1
2
3
4
5
6
7
8
9
10
11
12
2
3
4
5
6
7
8
9
10
11
12
对应的实现
package com.stt.yygh.order.service.impl;
import com.alibaba.fastjson.JSONObject;
import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper;
import com.baomidou.mybatisplus.extension.plugins.pagination.Page;
import com.baomidou.mybatisplus.extension.service.impl.ServiceImpl;
import com.stt.yygh.common.exception.YyghException;
import com.stt.yygh.common.helper.HttpRequestHelper;
import com.stt.yygh.common.result.ResultCodeEnum;
import com.stt.yygh.enums.OrderStatusEnum;
import com.stt.yygh.hosp.client.HospitalFeignClient;
import com.stt.yygh.model.order.OrderInfo;
import com.stt.yygh.model.user.Patient;
import com.stt.yygh.order.mapper.OrderInfoMapper;
import com.stt.yygh.order.service.OrderService;
import com.stt.yygh.rabbitmq.MqConst;
import com.stt.yygh.rabbitmq.RabbitService;
import com.stt.yygh.user.client.PatientFeignClient;
import com.stt.yygh.vo.o.OrderMqVo;
import com.stt.yygh.vo.hosp.ScheduleOrderVo;
import com.stt.yygh.vo.hosp.SignInfoVo;
import com.stt.yygh.vo.msm.MsmVo;
import com.stt.yygh.vo.order.OrderQueryVo;
import org.joda.time.DateTime;
import org.springframework.beans.BeanUtils;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import org.springframework.transaction.annotation.Transactional;
import org.springframework.util.StringUtils;
import java.util.HashMap;
import java.util.Map;
import java.util.Objects;
import java.util.Random;
@Service
public class OrderServiceImpl extends ServiceImpl<OrderInfoMapper, OrderInfo> implements OrderService {
...
//根据订单id查询订单详情
@Override
public OrderInfo getOrder(Long orderId) {
OrderInfo orderInfo = baseMapper.selectById(orderId);
return this.packOrderInfo(orderInfo);
}
...
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
# 添加controller
在 OrderApiController
添加接口
package com.stt.yygh.order.controller;
import com.baomidou.mybatisplus.extension.plugins.pagination.Page;
import com.stt.yygh.common.result.Result;
import com.stt.yygh.common.utils.AuthContextHolder;
import com.stt.yygh.enums.OrderStatusEnum;
import com.stt.yygh.model.order.OrderInfo;
import com.stt.yygh.order.service.OrderService;
import com.stt.yygh.vo.order.OrderQueryVo;
import io.swagger.annotations.Api;
import io.swagger.annotations.ApiOperation;
import io.swagger.annotations.ApiParam;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.*;
import javax.servlet.http.HttpServletRequest;
@Api(tags = "订单接口")
@RestController
@RequestMapping("/api/order/orderInfo")
public class OrderApiController {
@Autowired
private OrderService service;
...
//根据订单id查询订单详情
@GetMapping("auth/getOrders/{orderId}")
public Result getOrders(@PathVariable Long orderId) {
OrderInfo orderInfo = service.getOrder(orderId);
return Result.ok(orderInfo);
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
# 订单详情 yygh-site
# 封装api
在/api/order/orderInfo.js添加方法
import request from '@/utils/request'
const api_name = `/api/order/orderInfo`
...
//订单详情
export function getOrders(orderId) {
return request({
url: `${api_name}/auth/getOrders/${orderId}`,
method: `get`
})
}
1
2
3
4
5
6
7
8
9
10
11
2
3
4
5
6
7
8
9
10
11
# 创建页面组件
创建/pages/order/show.vue组件
<template>
<!-- header -->
<div class="nav-container page-component">
<!--左侧导航 #start -->
<div class="nav left-nav">
<div class="nav-item">
<span class="v-link clickable dark" onclick="javascript:window.location='/user'">实名认证</span>
</div>
<div class="nav-item selected">
<span class="v-link selected dark" onclick="javascript:window.location='/order'">挂号订单</span>
</div>
<div class="nav-item">
<span class="v-link clickable dark" onclick="javascript:window.location='/patient'">就诊人管理</span>
</div>
<div class="nav-item"><span class="v-link clickable dark">修改账号信息</span></div>
<div class="nav-item"><span class="v-link clickable dark">意见反馈</span></div>
</div>
<!-- 左侧导航 #end -->
<!-- 右侧内容 #start -->
<div class="page-container">
<div class="order-detail">
<div class="title"> 挂号详情</div>
<div class="status-bar">
<div class="left-wrapper">
<div class="status-wrapper BOOKING_SUCCESS">
<span class="iconfont"></span> {{ orderInfo.param.orderStatusString }}
</div>
</div>
<div class="right-wrapper">
<img src="//img.114yygh.com/static/web/code_order_detail.png" class="code-img">
<div class="content-wrapper">
<div> 微信<span class="iconfont"></span>关注“北京114预约挂号”</div>
<div class="watch-wrapper"> 快速挂号,轻松就医</div>
</div>
</div>
</div>
<div class="info-wrapper">
<div class="title-wrapper"><span class="block"></span><span>挂号信息</span></div>
<div class="info-form">
<el-form ref="form" :model="form">
<el-form-item label="就诊人信息:"><span class="content">{{ orderInfo.patientName }}</span></el-form-item>
<el-form-item label="就诊日期:">
<span class="content">{{ orderInfo.reserveDate }} {{ orderInfo.reserveTime == 0 ? '上午' : '下午' }}</span>
</el-form-item>
<el-form-item label="就诊医院:"><span class="content">{{ orderInfo.hosname }}</span></el-form-item>
<el-form-item label="就诊科室:"><span class="content">{{ orderInfo.depname }}</span></el-form-item>
<el-form-item label="医生职称:"><span class="content">{{ orderInfo.title }}</span></el-form-item>
<el-form-item label="医事服务费:"><span class="content fee">{{ orderInfo.amount }}元</span></el-form-item>
<el-form-item label="挂号单号:"><span class="content">{{ orderInfo.outTradeNo }}</span></el-form-item>
<el-form-item label="挂号时间:"><span class="content">{{ orderInfo.createTime }}</span></el-form-item>
</el-form>
</div>
</div>
<div class="rule-wrapper mt40">
<div class="rule-title"> 注意事项</div>
<div>1、请确认就诊人信息是否准确,若填写错误将无法取号就诊,损失由本人承担;<br>
<span style="color:red">2、【取号】就诊当天需在{{ orderInfo.fetchTime }}在医院取号,未取号视为爽约,该号不退不换;</span><br>
3、【退号】在{{ orderInfo.quitTime }}前可在线退号 ,逾期将不可办理退号退费;<br>
4、北京114预约挂号支持自费患者使用身份证预约,同时支持北京市医保患者使用北京社保卡在平台预约挂号。请于就诊当日,携带预约挂号所使用的有效身份证件到院取号;<br>
5、请注意北京市医保患者在住院期间不能使用社保卡在门诊取号。
</div>
</div>
<div class="bottom-wrapper mt60" v-if="orderInfo.orderStatus == 0 || orderInfo.orderStatus == 1">
<div class="button-wrapper">
<div class="v-button white" @click="cancelOrder()">取消预约</div>
</div>
<div class="button-wrapper ml20" v-if="orderInfo.orderStatus == 0">
<div class="v-button" @click="pay()">支付</div>
</div>
</div>
</div>
</div>
<!-- 右侧内容 #end -->
<!-- 微信支付弹出框 -->
<el-dialog :visible.sync="dialogPayVisible" style="text-align: left" :append-to-body="true" width="500px"
@close="closeDialog">
<div class="container">
<div class="operate-view" style="height: 350px;">
<div class="wrapper wechat">
<img src="images/weixin.jpg" alt="">
<span style="text-align: center;line-height: 25px;margin-bottom: 40px;">
请使用微信扫一扫<br/>扫描二维码支付
</span>
</div>
</div>
</div>
</el-dialog>
</div>
<!-- footer -->
</template>
<script>
import '~/assets/css/hospital_personal.css'
import '~/assets/css/hospital.css'
import { getOrders } from '@/api/order/orderInfo'
export default {
data() {
return {
orderId: null,
orderInfo: {
param: {}
},
dialogPayVisible: false,
payObj: {},
timer: null // 定时器名称
}
},
created() {
this.orderId = this.$route.query.orderId
this.init()
},
methods: {
init() {
getOrders(this.orderId).then(res => {
this.orderInfo = res.data
})
}
}
}
</script>
<style>
.info-wrapper {
padding-left: 0;
padding-top: 0;
}
.content-wrapper {
color: #333;
font-size: 14px;
padding-bottom: 0;
}
.bottom-wrapper {
width: 100%;
}
.button-wrapper {
margin: 0;
}
.bottom-wrapper .button-wrapper {
margin-top: 0;
}
</style>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
134
135
136
137
138
139
140
141
142
143
144
145
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
134
135
136
137
138
139
140
141
142
143
144
145
Last Updated: 2022/01/16, 11:29:51