27.前台系统-就诊人管理
# 需求分析
预约下单需要选择就诊人,因此要实现就诊人管理
用户下可以挂载多个就诊人信息,帮助他们进行挂号
就诊人管理其实就是要实现一个完整的增删改查
添加就诊人页面
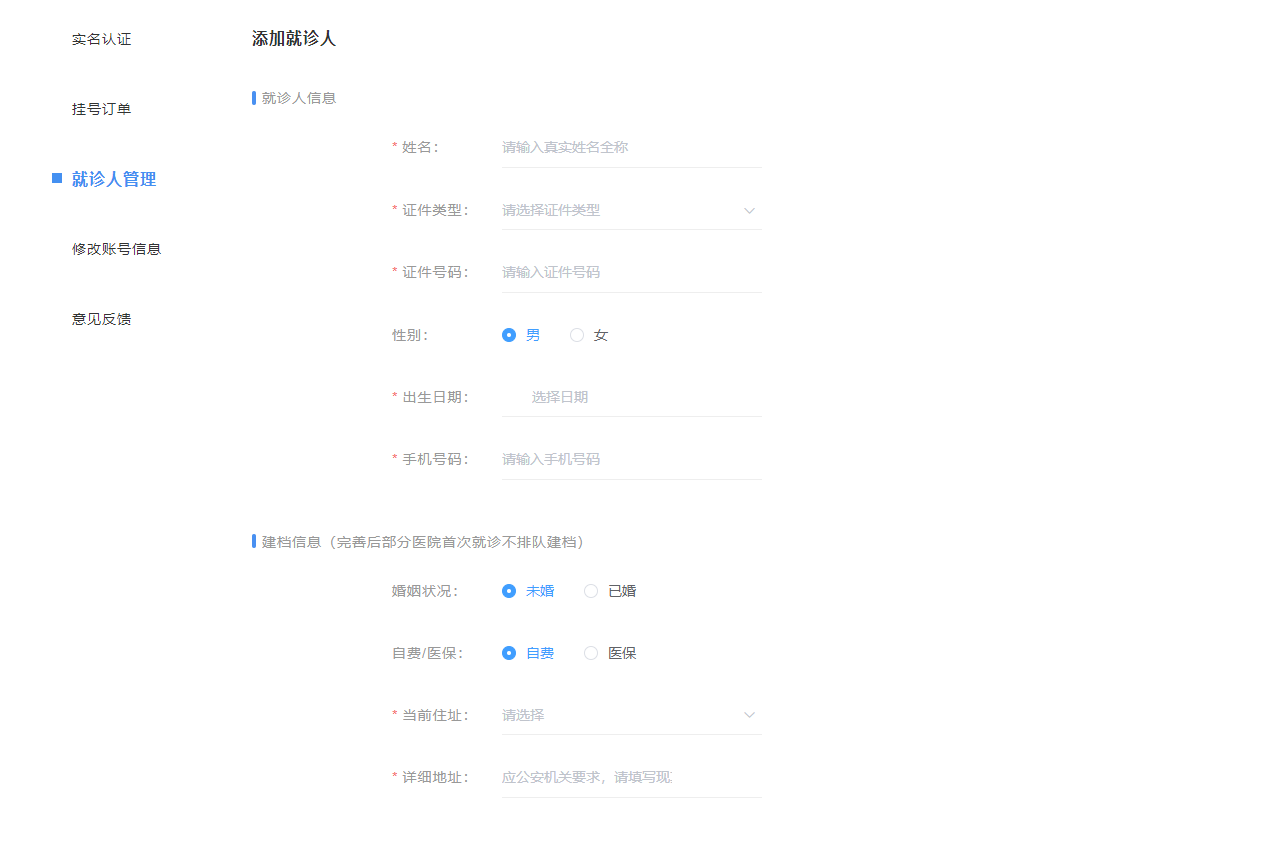
就诊人管理列表页面
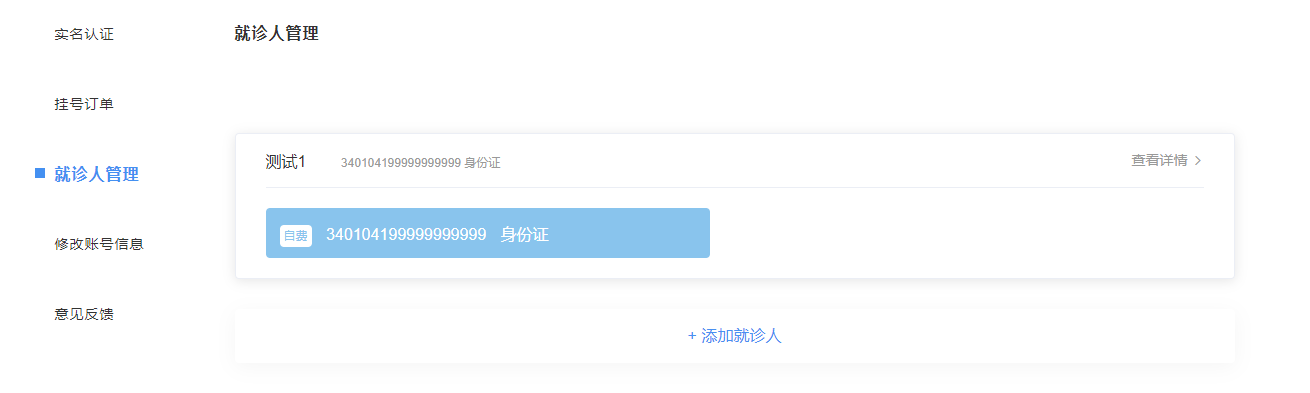
就诊人详情页面
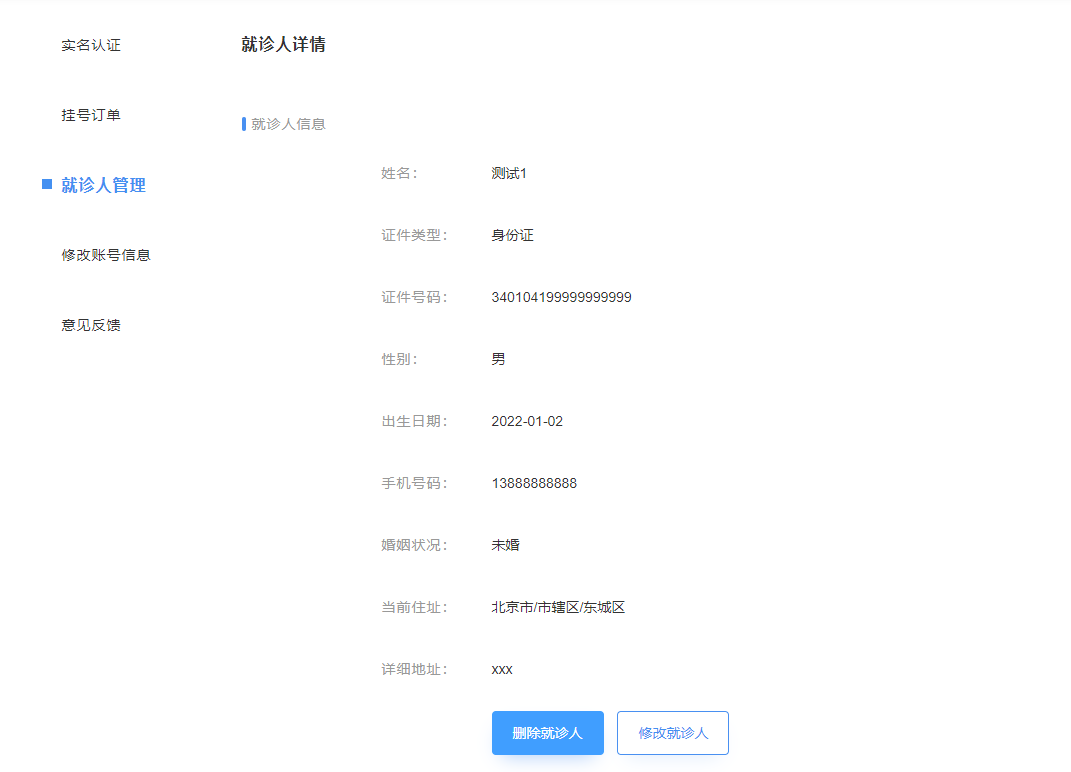
# 就诊人管理 service-user
# 修改pom引入依赖
引入该依赖,后续操作需要查询字典表数据
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<parent>
<artifactId>service</artifactId>
<groupId>com.stt.yygh</groupId>
<version>0.0.1</version>
</parent>
...
<dependencies>
<dependency>
<groupId>com.stt.yygh</groupId>
<artifactId>service-cmn-client</artifactId>
<version>1.0</version>
</dependency>
</dependencies>
</project>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
# 添加-就诊人实体类 patient
在 module
模块的com.stt.yygh.model.user
中创建 patient 类,与数据库字段相对应
package com.stt.yygh.model.user;
import com.baomidou.mybatisplus.annotation.TableField;
import com.baomidou.mybatisplus.annotation.TableName;
import com.fasterxml.jackson.annotation.JsonFormat;
import com.stt.yygh.model.base.BaseEntity;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import lombok.Data;
import java.util.Date;
@Data
@ApiModel(description = "Patient")
@TableName("patient")
public class patient extends BaseEntity {
private static final long serialVersionUID = 1L;
@ApiModelProperty(value = "用户id")
@TableField("user_id")
private Long userId;
@ApiModelProperty(value = "姓名")
@TableField("name")
private String name;
@ApiModelProperty(value = "证件类型")
@TableField("certificates_type")
private String certificatesType;
@ApiModelProperty(value = "证件编号")
@TableField("certificates_no")
private String certificatesNo;
@ApiModelProperty(value = "性别")
@TableField("sex")
private Integer sex;
@ApiModelProperty(value = "出生年月")
@JsonFormat(pattern = "yyyy-MM-dd")
@TableField("birthdate")
private Date birthdate;
@ApiModelProperty(value = "手机")
@TableField("phone")
private String phone;
@ApiModelProperty(value = "是否结婚")
@TableField("is_marry")
private Integer isMarry;
@ApiModelProperty(value = "省code")
@TableField("province_code")
private String provinceCode;
@ApiModelProperty(value = "市code")
@TableField("city_code")
private String cityCode;
@ApiModelProperty(value = "区code")
@TableField("district_code")
private String districtCode;
@ApiModelProperty(value = "详情地址")
@TableField("address")
private String address;
@ApiModelProperty(value = "联系人姓名")
@TableField("contacts_name")
private String contactsName;
@ApiModelProperty(value = "联系人证件类型")
@TableField("contacts_certificates_type")
private String contactsCertificatesType;
@ApiModelProperty(value = "联系人证件号")
@TableField("contacts_certificates_no")
private String contactsCertificatesNo;
@ApiModelProperty(value = "联系人手机")
@TableField("contacts_phone")
private String contactsPhone;
@ApiModelProperty(value = "是否有医保")
@TableField("is_insure")
private Integer isInsure;
@ApiModelProperty(value = "就诊卡")
@TableField("card_no")
private String cardNo;
@ApiModelProperty(value = "状态(0:默认 1:已认证)")
@TableField("status")
private String status;
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
# 添加-就诊人 mapper
在service-user
中添加 com.stt.yygh.user.mapper.PatientMapper
package com.stt.yygh.user.mapper;
import com.baomidou.mybatisplus.core.mapper.BaseMapper;
import com.stt.yygh.model.user.Patient;
import org.apache.ibatis.annotations.Mapper;
@Mapper
public interface PatientMapper extends BaseMapper<Patient> {
}
1
2
3
4
5
6
7
8
9
2
3
4
5
6
7
8
9
在com.stt.yygh.user.mapper.xml
中添加 PatientMapper.xml
文件
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.stt.yygh.user.mapper.PatientMapper">
</mapper>
1
2
3
4
5
6
2
3
4
5
6
# 添加-就诊人 service
添加com.stt.yygh.user.service.PatientService
接口
package com.stt.yygh.user.service;
import com.baomidou.mybatisplus.extension.service.IService;
import com.stt.yygh.model.user.Patient;
import java.util.List;
public interface PatientService extends IService<Patient> {
//获取就诊人列表
List<Patient> findAllUserId(Long userId);
//根据id获取就诊人信息
Patient getPatientId(Long id);
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
2
3
4
5
6
7
8
9
10
11
12
13
14
15
添加com.stt.yygh.user.service.impl.PatientServiceImpl
接口实现
package com.stt.yygh.user.service.impl;
import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper;
import com.baomidou.mybatisplus.extension.service.impl.ServiceImpl;
import com.stt.yygh.cmn.client.DictFeignClient;
import com.stt.yygh.enums.DictEnum;
import com.stt.yygh.model.user.Patient;
import com.stt.yygh.user.mapper.PatientMapper;
import com.stt.yygh.user.service.PatientService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.util.List;
@Service
public class PatientServiceImpl extends ServiceImpl<PatientMapper, Patient> implements PatientService {
@Autowired
private DictFeignClient dictFeignClient;
//获取就诊人列表
@Override
public List<Patient> findAllUserId(Long userId) {
//根据userid查询所有就诊人信息列表
QueryWrapper<Patient> wrapper = new QueryWrapper<>();
wrapper.eq("user_id",userId);
List<Patient> patientList = baseMapper.selectList(wrapper);
//通过远程调用,得到编码对应具体内容,查询数据字典表内容
//其他参数封装
patientList.forEach(this::packPatient);
return patientList;
}
@Override
public Patient getPatientId(Long id) {
return this.packPatient(baseMapper.selectById(id));
}
//Patient对象里面其他参数封装
private Patient packPatient(Patient patient) {
//根据证件类型编码,获取证件类型具体指
//就诊人证件类型
String certificatesTypeString = dictFeignClient.getName(DictEnum.CERTIFICATES_TYPE.getDictCode(), patient.getCertificatesType());
//联系人证件类型
String contactsCertificatesTypeString = dictFeignClient.getName(DictEnum.CERTIFICATES_TYPE.getDictCode(),patient.getContactsCertificatesType());
//省
String provinceString = dictFeignClient.getName(patient.getProvinceCode());
//市
String cityString = dictFeignClient.getName(patient.getCityCode());
//区
String districtString = dictFeignClient.getName(patient.getDistrictCode());
patient.getParam().put("certificatesTypeString", certificatesTypeString);
patient.getParam().put("contactsCertificatesTypeString", contactsCertificatesTypeString);
patient.getParam().put("provinceString", provinceString);
patient.getParam().put("cityString", cityString);
patient.getParam().put("districtString", districtString);
patient.getParam().put("fullAddress", provinceString + cityString + districtString + patient.getAddress());
return patient;
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
# 添加-就诊人 controller
添加 com.stt.yygh.user.api.PatientApiController
包含了就诊人的增删改查操作
package com.stt.yygh.user.controller;
import com.stt.yygh.common.result.Result;
import com.stt.yygh.common.utils.AuthContextHolder;
import com.stt.yygh.model.user.Patient;
import com.stt.yygh.user.service.PatientService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.*;
import javax.servlet.http.HttpServletRequest;
import java.util.List;
//就诊人管理接口
@RestController
@RequestMapping("/api/user/patient")
public class PatientApiController {
@Autowired
private PatientService service;
//获取就诊人列表
@GetMapping("auth/findAll")
public Result findAll(HttpServletRequest request) {
//获取当前登录用户id
Long userId = AuthContextHolder.getUserId(request);
List<Patient> list = service.findAllUserId(userId);
return Result.ok(list);
}
//添加就诊人
@PostMapping("auth/save")
public Result savePatient(@RequestBody Patient patient, HttpServletRequest request) {
//获取当前登录用户id
Long userId = AuthContextHolder.getUserId(request);
patient.setUserId(userId);
service.save(patient);
return Result.ok();
}
//根据id获取就诊人信息
@GetMapping("auth/get/{id}")
public Result getPatient(@PathVariable Long id) {
Patient patient = service.getPatientId(id);
return Result.ok(patient);
}
//修改就诊人
@PostMapping("auth/update")
public Result updatePatient(@RequestBody Patient patient) {
service.updateById(patient);
return Result.ok();
}
//删除就诊人
@DeleteMapping("auth/remove/{id}")
public Result removePatient(@PathVariable Long id) {
service.removeById(id);
return Result.ok();
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
# 就诊人管理 yygh-site
# 封装api接口
创建/api/user/patient.js文件
import request from '@/utils/request'
const api_name = `/api/user/patient`
//就诊人列表
export function findList() {
return request({
url: `${api_name}/auth/findAll`,
method: `get`
})
}
//根据id查询就诊人信息
export function getById(id) {
return request({
url: `${api_name}/auth/get/${id}`,
method: 'get'
})
}
//添加就诊人信息
export function save(patient) {
return request({
url: `${api_name}/auth/save`,
method: 'post',
data: patient
})
}
//修改就诊人信息
export function updateById(patient) {
return request({
url: `${api_name}/auth/update`,
method: 'post',
data: patient
})
}
//删除就诊人信息
export function removeById(id) {
return request({
url: `${api_name}/auth/remove/${id}`,
method: 'delete'
})
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
注意:在service-gateway中对 /api/**/auth/**
接口进行了拦截,针对该类型的接口,进行了登录验证
# 创建-就诊人列表页面
添加 /pages/patient/index.vue
组件
<template>
<!-- header -->
<div class="nav-container page-component">
<!--左侧导航 #start -->
<div class="nav left-nav">
<div class="nav-item "><span class="v-link clickable dark" @click="toPage('/user')">实名认证</span></div>
<div class="nav-item "><span class="v-link clickable dark" @click="toPage('/order')">挂号订单</span></div>
<div class="nav-item selected">
<span class="v-link selected dark" @click="toPage('/patient')">就诊人管理</span>
</div>
<div class="nav-item "><span class="v-link clickable dark">修改账号信息</span></div>
<div class="nav-item "><span class="v-link clickable dark">意见反馈</span></div>
</div>
<!-- 左侧导航 #end -->
<!-- 右侧内容 #start -->
<div class="page-container">
<div class="personal-patient">
<div class="header-wrapper">
<div class="title"> 就诊人管理</div>
</div>
<div class="content-wrapper">
<el-card class="patient-card" shadow="always" v-for="item in patientList" :key="item.id">
<div slot="header" class="clearfix">
<div>
<span class="name">{{ item.name }}</span>
<span>{{ item.certificatesNo }} {{ item.param.certificatesTypeString }}</span>
<div class="detail" @click="show(item.id)"> 查看详情 <span class="iconfont"></span></div>
</div>
</div>
<div class="card SELF_PAY_CARD">
<div class="info">
<span class="type">{{ item.isInsure == 0 ? '自费' : '医保' }}</span>
<span class="card-no">{{ item.certificatesNo }}</span>
<span class="card-view">{{ item.param.certificatesTypeString }}</span>
</div>
<span class="operate"></span>
</div>
<div class="card">
<div class="text bind-card"></div>
</div>
</el-card>
<div class="item-add-wrapper v-card clickable" @click="add()">
<div class="">
<div>+ 添加就诊人</div>
</div>
</div>
</div>
</div>
</div>
<!-- 右侧内容 #end -->
</div>
<!-- footer -->
</template>
<script>
import '~/assets/css/hospital_personal.css'
import '~/assets/css/hospital.css'
import '~/assets/css/personal.css'
import { findList } from '@/api/user/patient'
export default {
data() {
return {
patientList: []
}
},
created() {
this.findPatientList()
},
methods: {
findPatientList() {
findList().then(res => {
this.patientList = res.data
})
},
toPage(path) {
window.location = path
},
add() {
window.location.href = '/patient/add'
},
show(id) {
window.location.href = '/patient/show?id=' + id
}
}
}
</script>
<style>
.header-wrapper .title {
font-size: 16px;
margin-top: 0;
}
.content-wrapper {
margin-left: 0;
}
.patient-card .el-card__header .detail {
font-size: 14px;
}
</style>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
# 创建-添加和修改就诊人页面
添加/pages/patient/add.vue
组件
<template>
<!-- header -->
<div class="nav-container page-component">
<!--左侧导航 #start -->
<div class="nav left-nav">
<div class="nav-item "><span class="v-link clickable dark" @click="toPage('/user')">实名认证</span></div>
<div class="nav-item "><span class="v-link clickable dark" @click="toPage('/order')">挂号订单</span></div>
<div class="nav-item selected">
<span class="v-link selected dark" @click="toPage('/patient')">就诊人管理</span>
</div>
<div class="nav-item "><span class="v-link clickable dark">修改账号信息</span></div>
<div class="nav-item "><span class="v-link clickable dark">意见反馈</span></div>
</div>
<!-- 左侧导航 #end -->
<!-- 右侧内容 #start -->
<div class="page-container">
<div class="personal-patient">
<div class="header-wrapper">
<div class="title">添加就诊人</div>
</div>
<div>
<div class="sub-title">
<div class="block"></div>
就诊人信息
</div>
<div class="content-wrapper">
<el-form :model="patient" label-width="110px" label-position="left" ref="patient" :rules="validateRules">
<el-form-item prop="name" label="姓名:">
<el-input v-model="patient.name" placeholder="请输入真实姓名全称" class="input v-input"/>
</el-form-item>
<el-form-item prop="certificatesType" label="证件类型:">
<el-select v-model="patient.certificatesType" placeholder="请选择证件类型" class="v-select patient-select">
<el-option v-for="item in certificatesTypeList" :key="item.value"
:label="item.name" :value="item.value">
</el-option>
</el-select>
</el-form-item>
<el-form-item prop="certificatesNo" label="证件号码:">
<el-input v-model="patient.certificatesNo" placeholder="请输入证件号码" class="input v-input"/>
</el-form-item>
<el-form-item prop="sex" label="性别:">
<el-radio-group v-model="patient.sex">
<el-radio :label="1">男</el-radio>
<el-radio :label="0">女</el-radio>
</el-radio-group>
</el-form-item>
<el-form-item prop="birthdate" label="出生日期:">
<el-date-picker v-model="patient.birthdate" class="v-date-picker" type="date" placeholder="选择日期"/>
</el-form-item>
<el-form-item prop="phone" label="手机号码:">
<el-input v-model="patient.phone" placeholder="请输入手机号码" maxlength="11" class="input v-input"/>
</el-form-item>
</el-form>
</div>
<div class="sub-title">
<div class="block"></div>
建档信息(完善后部分医院首次就诊不排队建档)
</div>
<div class="content-wrapper">
<el-form :model="patient" label-width="110px" label-position="left" ref="patient" :rules="validateRules">
<el-form-item prop="isMarry" label="婚姻状况:">
<el-radio-group v-model="patient.isMarry">
<el-radio :label="0">未婚</el-radio>
<el-radio :label="1">已婚</el-radio>
</el-radio-group>
</el-form-item>
<el-form-item prop="isInsure" label="自费/医保:">
<el-radio-group v-model="patient.isInsure">
<el-radio :label="0">自费</el-radio>
<el-radio :label="1">医保</el-radio>
</el-radio-group>
</el-form-item>
<el-form-item prop="addressSelected" label="当前住址:">
<el-cascader ref="selectedShow" v-model="patient.addressSelected" class="v-address" :props="props"/>
</el-form-item>
<el-form-item prop="address" label="详细地址:">
<el-input v-model="patient.address" placeholder="应公安机关要求,请填写现真实住址" class="input v-input"/>
</el-form-item>
</el-form>
</div>
<div class="sub-title">
<div class="block"></div>
联系人信息(选填)
</div>
<div class="content-wrapper">
<el-form :model="patient" label-width="110px" label-position="left">
<el-form-item prop="contactsName" label="姓名:">
<el-input v-model="patient.contactsName" placeholder="请输入联系人姓名全称" class="input v-input"/>
</el-form-item>
<el-form-item prop="contactsCertificatesType" label="证件类型:">
<el-select v-model="patient.contactsCertificatesType" placeholder="请选择证件类型"
class="v-select patient-select">
<el-option v-for="item in certificatesTypeList" :key="item.value"
:label="item.name" :value="item.value">
</el-option>
</el-select>
</el-form-item>
<el-form-item prop="contactsCertificatesNo" label="证件号码:">
<el-input v-model="patient.contactsCertificatesNo" placeholder="请输入联系人证件号码" class="input v-input"/>
</el-form-item>
<el-form-item prop="contactsPhone" label="手机号码:">
<el-input v-model="patient.contactsPhone" placeholder="请输入联系人手机号码" class="input v-input"/>
</el-form-item>
</el-form>
</div>
</div>
<div class="bottom-wrapper">
<div class="button-wrapper">
<div class="v-button" @click="saveOrUpdate()">{{ submitBnt }}</div>
</div>
</div>
</div>
</div>
<!-- 右侧内容 #end -->
</div>
<!-- footer -->
</template>
<script>
import '~/assets/css/hospital_personal.css'
import '~/assets/css/hospital.css'
import '~/assets/css/personal.css'
import { findByDictCode, findByParentId } from '@/api/cmn/dict'
import { getById, save, updateById } from '@/api/user/patient'
const defaultForm = {
name: '',
certificatesType: '',
certificatesNo: '',
sex: 1,
birthdate: '',
phone: '',
isMarry: 0,
isInsure: 0,
provinceCode: '',
cityCode: '',
districtCode: '',
addressSelected: null,
address: '',
contactsName: '',
contactsCertificatesType: '',
contactsCertificatesNo: '',
contactsPhone: '',
param: {}
}
export default {
data() {
return {
patient: defaultForm,
certificatesTypeList: [],
props: {
lazy: true,
async lazyLoad(node, resolve) {
const { level } = node
//异步获取省市区
findByParentId(level ? node.value : '86').then(res => {
let list = res.data
let provinceList = list.map((item, i) => {
return {
value: item.id,
label: item.name,
leaf: node.level === 2 //可控制显示几级
}
})
resolve && resolve(provinceList)
})
}
},
submitBnt: '保存',
validateRules: {
name: [{ required: true, trigger: 'blur', message: '必须输入' }],
certificatesType: [{ required: true, trigger: 'blur', message: '必须输入' }],
certificatesNo: [{ required: true, trigger: 'blur', message: '必须输入' }],
birthdate: [{ required: true, trigger: 'blur', message: '必须输入' }],
phone: [{ required: true, trigger: 'blur', message: '必须输入' }],
addressSelected: [{ required: true, trigger: 'blur', message: '必须输入' }],
address: [{ required: true, trigger: 'blur', message: '必须输入' }]
}
}
},
created() {
this.init()
},
mounted() {
if (this.$route.query.id) {
setTimeout(() => {
//"北京市/市辖区/西城区";// 首次手动复制
this.$refs.selectedShow.presentText = this.patient.param.provinceString + '/' + this.patient.param.cityString + '/' + this.patient.param.districtString
}, 1000)
}
},
methods: {
toPage(path) {
window.location = path
},
init() {
if (this.$route.query.id) {
const id = this.$route.query.id
this.fetchDataById(id)
} else {
// 对象拓展运算符:拷贝对象,而不是赋值对象的引用
this.patient = { ...defaultForm }
}
this.getDict()
},
fetchDataById(id) {
getById(id).then(res => {
this.patient = res.data
//添加默认值
this.patient.addressSelected = [this.patient.provinceCode, this.patient.cityCode, this.patient.districtCode]
})
},
getDict() {
findByDictCode('CertificatesType').then(res => {
this.certificatesTypeList = res.data
})
},
saveOrUpdate() {
this.$refs.patient.validate(valid => {
if (valid) {
//地址处理
if (this.patient.addressSelected.length === 3) {
this.patient.provinceCode = this.patient.addressSelected[0]
this.patient.cityCode = this.patient.addressSelected[1]
this.patient.districtCode = this.patient.addressSelected[2]
}
if (!this.patient.id) {
this.saveData()
} else {
this.updateData()
}
}
})
},
// 新增
saveData() {
if (this.submitBnt === '正在提交...') {
this.$message.info('重复提交')
return
}
this.submitBnt = '正在提交...'
save(this.patient).then(res => {
this.$message.success('提交成功')
window.location.href = '/patient'
}).catch(e => {
this.submitBnt = '保存'
})
},
// 根据id更新记录
updateData() {
if (this.submitBnt === '正在提交...') {
this.$message.info('重复提交')
return
}
this.submitBnt = '正在提交...'
updateById(this.patient).then(response => {
this.$message.success('提交成功')
window.location.href = '/patient'
}).catch(e => {
this.submitBnt = '保存'
})
}
}
}
</script>
<style>
.header-wrapper .title {
font-size: 16px;
margin-top: 0;
}
.sub-title {
margin-top: 0;
}
.bottom-wrapper {
padding: 0;
margin: 0;
}
.bottom-wrapper .button-wrapper {
margin-top: 0;
}
</style>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
134
135
136
137
138
139
140
141
142
143
144
145
146
147
148
149
150
151
152
153
154
155
156
157
158
159
160
161
162
163
164
165
166
167
168
169
170
171
172
173
174
175
176
177
178
179
180
181
182
183
184
185
186
187
188
189
190
191
192
193
194
195
196
197
198
199
200
201
202
203
204
205
206
207
208
209
210
211
212
213
214
215
216
217
218
219
220
221
222
223
224
225
226
227
228
229
230
231
232
233
234
235
236
237
238
239
240
241
242
243
244
245
246
247
248
249
250
251
252
253
254
255
256
257
258
259
260
261
262
263
264
265
266
267
268
269
270
271
272
273
274
275
276
277
278
279
280
281
282
283
284
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
134
135
136
137
138
139
140
141
142
143
144
145
146
147
148
149
150
151
152
153
154
155
156
157
158
159
160
161
162
163
164
165
166
167
168
169
170
171
172
173
174
175
176
177
178
179
180
181
182
183
184
185
186
187
188
189
190
191
192
193
194
195
196
197
198
199
200
201
202
203
204
205
206
207
208
209
210
211
212
213
214
215
216
217
218
219
220
221
222
223
224
225
226
227
228
229
230
231
232
233
234
235
236
237
238
239
240
241
242
243
244
245
246
247
248
249
250
251
252
253
254
255
256
257
258
259
260
261
262
263
264
265
266
267
268
269
270
271
272
273
274
275
276
277
278
279
280
281
282
283
284
# 创建-就诊人详情页面(含删除功能)
添加/pages/patient/show.vue
组件
<template>
<!-- header -->
<div class="nav-container page-component">
<!--左侧导航 #start -->
<div class="nav left-nav">
<div class="nav-item "><span class="v-link clickable dark" @click="toPage('/user')">实名认证</span></div>
<div class="nav-item "><span class="v-link clickable dark" @click="toPage('/order')">挂号订单</span></div>
<div class="nav-item selected">
<span class="v-link selected dark" @click="toPage('/patient')">就诊人管理</span>
</div>
<div class="nav-item "><span class="v-link clickable dark">修改账号信息</span></div>
<div class="nav-item "><span class="v-link clickable dark">意见反馈</span></div>
</div>
<!-- 右侧内容 #start -->
<div class="page-container">
<div class="personal-patient">
<div class="title" style="margin-top: 0;font-size: 16px;">就诊人详情</div>
<div>
<div class="sub-title"><i class="block"></i>就诊人信息</div>
<div class="content-wrapper">
<el-form :model="patient" label-width="110px" label-position="left">
<el-form-item label="姓名:">{{ patient.name }}</el-form-item>
<el-form-item label="证件类型:">{{ patient.param.certificatesTypeString }}</el-form-item>
<el-form-item label="证件号码:">{{ patient.certificatesNo }}</el-form-item>
<el-form-item label="性别:">{{ patient.sex == 1 ? '男' : '女' }}</el-form-item>
<el-form-item label="出生日期:">{{ patient.birthdate }}</el-form-item>
<el-form-item label="手机号码:">{{ patient.phone }}</el-form-item>
<el-form-item label="婚姻状况:">{{ patient.isMarry == 1 ? '已婚' : '未婚' }}</el-form-item>
<el-form-item label="当前住址:">
{{ patient.param.provinceString }}/{{ patient.param.cityString }}/{{ patient.param.districtString }}
</el-form-item>
<el-form-item label="详细地址:">{{ patient.address }}</el-form-item>
<el-form-item>
<el-button class="v-button" type="primary" @click="remove()">删除就诊人</el-button>
<el-button class="v-button" type="primary white" @click="edit()">修改就诊人</el-button>
</el-form-item>
</el-form>
</div>
</div>
</div>
</div><!-- 右侧内容 #end -->
</div><!-- footer -->
</template>
<script>
import '~/assets/css/hospital_personal.css'
import '~/assets/css/hospital.css'
import '~/assets/css/personal.css'
import { getById, removeById } from '@/api/user/patient'
export default {
data() {
return {
patient: {
param: {}
}
}
},
created() {
this.fetchDataById()
},
methods: {
toPage(path) {
window.location = path
},
fetchDataById() {
getById(this.$route.query.id).then(res => {
this.patient = res.data
})
},
remove() {
removeById(this.patient.id).then(res => {
this.$message.success('删除成功')
window.location.href = '/patient'
})
},
edit() {
window.location.href = '/patient/add?id=' + this.patient.id
}
}
}
</script>
<style>
.content-wrapper {
color: #333;
font-size: 14px;
padding-bottom: 0;
}
</style>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
Last Updated: 2022/01/16, 11:29:51