17.后台系统-排班管理
# 页面效果
点击医院管理中一条记录的【排班】操作按键,进入到排班管理页面
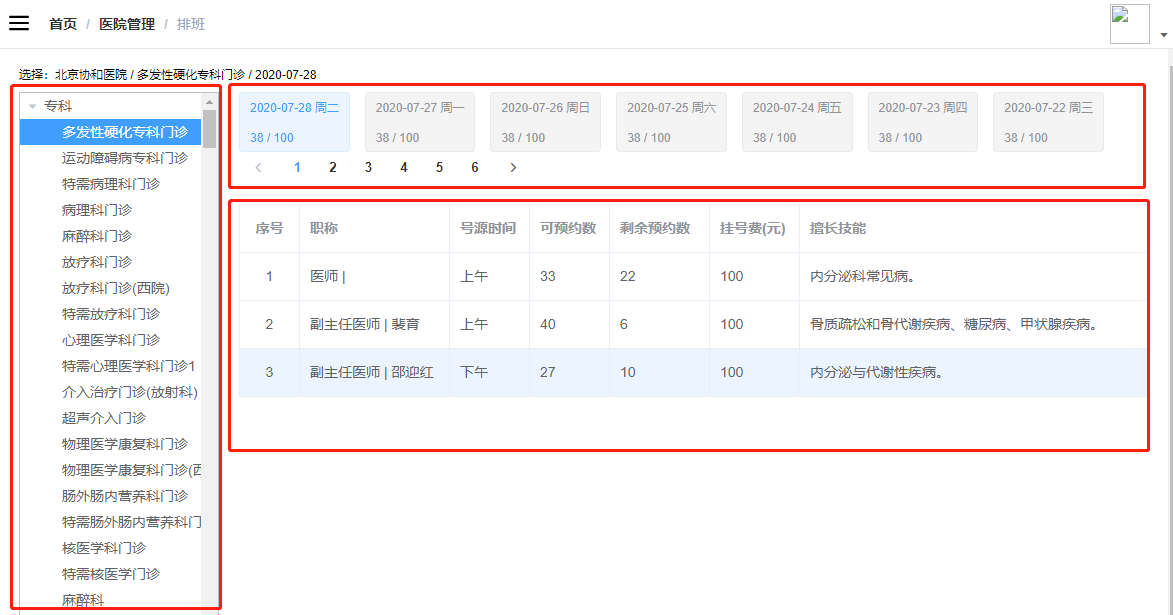
排班分成三部分显示
- 科室信息(大科室与小科室树形展示)
- 排班日期,分页显示,根据上传排班数据聚合统计产生
- 排班日期对应的就诊医生信息
# 接口分析
- 科室数据使用Element-ui el-tree组件渲染展示,需要将医院上传的科室数据封装成两层父子级数据
- 聚合所有排班数据,按日期分页展示,并统计号源数据展示
- 根据排班日期获取排班详情数据
# 实现分析
虽然是一个页面展示所有内容,但是页面相对复杂,分步骤实现
- 先实现左侧科室树形展示
- 其次排班日期分页展示
- 最后根据排班日期获取排班详情数据
# 科室列表后端实现
# 数据分析
把所有科室按照树形结构显示,需要从mongo库中获取的数据解析成如下格式
[
{
bigcode:'1',
depname: '内科',
children: [
{
bigcode: '11',
depname: '普通内科'
}
...
]
}
...
]
2
3
4
5
6
7
8
9
10
11
12
13
14
# model中添加vo类
在model
中添加 DepartmentVo
类
package com.stt.yygh.vo.hosp;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import lombok.Data;
import java.util.List;
@Data
@ApiModel(description = "Department")
public class DepartmentVo {
@ApiModelProperty(value = "科室编号")
private String depcode;
@ApiModelProperty(value = "科室名称")
private String depname;
@ApiModelProperty(value = "下级节点")
private List<DepartmentVo> children;
}
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
# 添加controller
在service-hosp
创建DepartmentController
并添加接口方法
package com.stt.yygh.hosp.controller;
import com.stt.yygh.common.result.Result;
import com.stt.yygh.hosp.service.DepartmentService;
import com.stt.yygh.vo.hosp.DepartmentVo;
import io.swagger.annotations.ApiOperation;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.*;
import java.util.List;
@RestController
@RequestMapping("/admin/hosp/department")
@CrossOrigin
public class DepartmentController {
@Autowired
private DepartmentService service;
//根据医院编号,查询医院所有科室列表
@ApiOperation(value = "查询医院所有科室列表")
@GetMapping("getDeptList/{hoscode}")
public Result getDeptList(@PathVariable String hoscode) {
List<DepartmentVo> list = service.findDeptTree(hoscode);
return Result.ok(list);
}
}
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
# 添加service接口方法与实现
在service-hosp
的DepartmentService
中添加接口方法
package com.stt.yygh.hosp.service;
...
public interface DepartmentService {
...
List<DepartmentVo> findDeptTree(String hoscode);
}
2
3
4
5
6
在service-hosp
的DepartmentServiceImpl
中添加接口实现
package com.stt.yygh.hosp.service.impl;
...
@Service
public class DepartmentServiceImpl implements DepartmentService {
...
@Override
public List<DepartmentVo> findDeptTree(String hoscode) {
// 通过医院编号查询所有科室信息
Department d = new Department();
d.setHoscode(hoscode);
List<Department> all = repository.findAll(Example.of(d));
// 通过科室bigcode进行分组
Map<String, List<Department>> departmentMap = all.stream()
.collect(Collectors.groupingBy(Department::getBigcode));
List<DepartmentVo> re = new ArrayList<>();
for (Map.Entry<String, List<Department>> entry : departmentMap.entrySet()) {
// 大科室编号
String bigcode = entry.getKey();
// 封装大科室输出对象
DepartmentVo parent = new DepartmentVo();
parent.setDepcode(bigcode);
parent.setDepname(entry.getValue().get(0).getDepname());
parent.setChildren(new ArrayList<>());
// 封装小科室
for (Department department : entry.getValue()) {
DepartmentVo subVo = new DepartmentVo();
subVo.setDepname(department.getDepname());
subVo.setDepcode(department.getDepcode());
parent.getChildren().add(subVo);
}
re.add(parent);
}
return re;
}
}
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
# 科室列表前端实现
# 添加路由
在 src/router/index.js中添加路由信息
{
path: '/hospSet',
component: Layout,
redirect: '/hospSet/list',
name: '医院设置管理',
meta: { title: '医院设置管理', icon: 'el-icon-s-help' },
children: [
...
{
path: 'hosp/schedule/:hoscode',
name: '排班',
component: () => import('@/views/hosp/schedule'),
meta: { title: '排班', noCache: true },
hidden: true
}
]
},
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
# 添加按钮
在 views/hosp/list.vue中添加按键,跳转到排班页面
<template>
<div class="app-container">
...
<el-table v-loading="listLoading" :data="list" border fit highlight-current-row>
...
<el-table-column label="操作" width="230" align="center">
<template slot-scope="scope">
...
<router-link :to="'/hospSet/hosp/schedule/'+scope.row.hoscode">
<el-button type="primary" size="mini">排班</el-button>
</router-link>
</template>
</el-table-column>
</el-table>
...
</div>
</template>
...
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
# 封装api
在 src/api/hosp.js中添加如下接口
// 查看部门列表
export function getDeptList(hoscode) {
return request({
url: `/admin/hosp/department/getDeptList/${hoscode}`,
method: 'get'
})
}
2
3
4
5
6
7
# 创建schedule.vue组件
创建/views/hosp/schedule.vue组件
<template>
<div class="app-container">
<div style="margin-bottom: 10px;font-size: 10px;">选择:</div>
<el-container style="height: 100%">
<el-aside width="200px" style="border: 1px silver solid">
<!-- 部门 -->
<el-tree :data="data" :props="defaultProps" :default-expand-all="true" @node-click="handleNodeClick"/>
</el-aside>
<el-main style="padding: 0 0 0 20px;">
<!-- 排班日期 分页 -->
<el-row style="width: 100%"></el-row>
<!-- 排班日期对应的排班医生 -->
<el-row style="margin-top: 20px;"></el-row>
</el-main>
</el-container>
</div>
</template>
<script>
import { getDeptList } from '@/api/hosp'
export default {
data() {
return {
data: [],
defaultProps: {
children: 'children',
label: 'depname'
},
hoscode: null
}
},
created() {
this.hoscode = this.$route.params.hoscode
this.fetchData()
},
methods: {
fetchData(deptList = getDeptList) {
deptList(this.hoscode)
.then(response => {
this.data = response.data
})
},
handleNodeClick() {
// 待完善
}
}
}
</script>
<style scoped>
.el-tree-node.is-current > .el-tree-node__content {
background-color: #409EFF !important;
color: white;
}
.el-checkbox__input.is-checked + .el-checkbox__label {
color: black;
}
</style>
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
底部style标签是为了控制树形展示数据选中效果的
# 排班日期分页列表后端实现
通过医院的编号和科室的编号查出排班信息
# model中添加vo类
在model
中添加 BookingScheduleRuleVo
类
package com.stt.yygh.vo.hosp;
import com.fasterxml.jackson.annotation.JsonFormat;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import lombok.Data;
import java.util.Date;
@Data
@ApiModel(description = "可预约排班规则数据")
public class BookingScheduleRuleVo {
@ApiModelProperty(value = "可预约日期")
@JsonFormat(pattern = "yyyy-MM-dd")
private Date workDate;
@ApiModelProperty(value = "可预约日期")
@JsonFormat(pattern = "MM月dd日")
private Date workDateMd; //方便页面使用
@ApiModelProperty(value = "周几")
private String dayOfWeek;
@ApiModelProperty(value = "就诊医生人数")
private Integer docCount;
@ApiModelProperty(value = "科室可预约数")
private Integer reservedNumber;
@ApiModelProperty(value = "科室剩余预约数")
private Integer availableNumber;
@ApiModelProperty(value = "状态 0:正常 1:即将放号 -1:当天已停止挂号")
private Integer status;
}
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
# 添加controller
在service-hosp
创建ScheduleController
并添加接口方法
package com.stt.yygh.hosp.controller;
import com.stt.yygh.common.result.Result;
import com.stt.yygh.hosp.service.ScheduleService;
import io.swagger.annotations.ApiOperation;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.*;
import java.util.Map;
@RestController
@RequestMapping("/admin/hosp/schedule")
@CrossOrigin
public class ScheduleController {
@Autowired
private ScheduleService service;
//根据医院编号 和 科室编号 ,查询排班规则数据
@ApiOperation(value = "查询排班规则数据")
@GetMapping("getScheduleRule/{page}/{limit}/{hoscode}/{depcode}")
public Result getScheduleRule(@PathVariable long page,
@PathVariable long limit,
@PathVariable String hoscode,
@PathVariable String depcode) {
Map<String, Object> map =
service.getRuleSchedule(page, limit, hoscode, depcode);
return Result.ok(map);
}
}
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
# 添加service
在service-hosp
的ScheduleService
中添加接口方法
package com.stt.yygh.hosp.service;
...
public interface ScheduleService {
...
Map<String, Object> getRuleSchedule(long page, long limit, String hoscode, String depcode);
}
2
3
4
5
6
在service-hosp
的ScheduleServiceImpl
中添加接口方法
package com.stt.yygh.hosp.service.impl;
import com.alibaba.fastjson.JSONObject;
import com.stt.yygh.hosp.repository.ScheduleRepository;
import com.stt.yygh.hosp.service.HospitalService;
import com.stt.yygh.hosp.service.ScheduleService;
import com.stt.yygh.model.hosp.Schedule;
import com.stt.yygh.vo.hosp.BookingScheduleRuleVo;
import com.stt.yygh.vo.hosp.ScheduleQueryVo;
import org.joda.time.DateTime;
import org.joda.time.DateTimeConstants;
import org.springframework.beans.BeanUtils;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.domain.*;
import org.springframework.data.mongodb.core.MongoTemplate;
import org.springframework.data.mongodb.core.aggregation.Aggregation;
import org.springframework.data.mongodb.core.aggregation.AggregationResults;
import org.springframework.data.mongodb.core.query.Criteria;
import org.springframework.stereotype.Service;
import java.util.*;
@Service
public class ScheduleServiceImpl implements ScheduleService {
@Autowired
private ScheduleRepository repository;
@Autowired
private MongoTemplate mongoTemplate;
@Autowired
private HospitalService hospitalService;
...
//根据医院编号 和 科室编号 ,查询排班规则数据
@Override
public Map<String, Object> getRuleSchedule(long page, long limit, String hoscode, String depcode) {
// 依据医院编号,科室编号查询
Criteria criteria = Criteria.where("hoscode").is(hoscode)
.and("depcode").is(depcode);
// 依据工作日进行分组
Aggregation agg = Aggregation.newAggregation(
Aggregation.match(criteria), // 匹配条件
Aggregation.group("workDate") // 分组字段
.first("workDate").as("workDate") // 根据资源文档的排序获取第一个文档数据
.count().as("docCount") // 统计号源个数
.sum("reservedNumber").as("reservedNumber")
.sum("availableNumber").as("availableNumber"),
Aggregation.sort(Sort.Direction.DESC, "workDate"),// 排序
Aggregation.skip((page - 1) * limit), // 分页
Aggregation.limit(limit)
);
AggregationResults<BookingScheduleRuleVo> aggResults = mongoTemplate.aggregate(agg,
Schedule.class, BookingScheduleRuleVo.class);
List<BookingScheduleRuleVo> bookingScheduleRuleVoList = aggResults.getMappedResults();
// 分页查询的总记录数
Aggregation totalAgg = Aggregation.newAggregation(
Aggregation.match(criteria),
Aggregation.group("workDate")
);
AggregationResults<BookingScheduleRuleVo> totalAggResults = mongoTemplate.aggregate(totalAgg,
Schedule.class, BookingScheduleRuleVo.class);
int total = totalAggResults.getMappedResults().size();
// 将日期对应的星期获取
for (BookingScheduleRuleVo vo : bookingScheduleRuleVoList) {
Date workDate = vo.getWorkDate();
String dayOfWeek = this.getDayOfWeek(new DateTime(workDate));
vo.setDayOfWeek(dayOfWeek);
}
//设置最终数据,进行返回
Map<String, Object> re = new HashMap<>();
re.put("bookingScheduleRuleList", bookingScheduleRuleVoList);
re.put("total", total);
//获取医院名称
String hosName = hospitalService.getHospName(hoscode);
//其他基础数据
Map<String, String> baseMap = new HashMap<>();
baseMap.put("hosname", hosName);
re.put("baseMap", baseMap);
return re;
}
private String getDayOfWeek(DateTime dateTime) {
Map<Integer, String> dayOfWeekMap = new HashMap<>();
dayOfWeekMap.put(DateTimeConstants.MONDAY, "周一");
dayOfWeekMap.put(DateTimeConstants.TUESDAY, "周二");
dayOfWeekMap.put(DateTimeConstants.WEDNESDAY, "周三");
dayOfWeekMap.put(DateTimeConstants.THURSDAY, "周四");
dayOfWeekMap.put(DateTimeConstants.FRIDAY, "周五");
dayOfWeekMap.put(DateTimeConstants.SATURDAY, "周六");
dayOfWeekMap.put(DateTimeConstants.SUNDAY, "周日");
if (dayOfWeekMap.containsKey(dateTime.getDayOfWeek())) {
return dayOfWeekMap.get(dateTime.getDayOfWeek());
}
return "";
}
}
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
在hospitalService
中添加接口与实现类
package com.stt.yygh.hosp.service;
...
public interface HospitalService {
...
String getHospName(String hoscode);
}
2
3
4
5
6
在HospitalServiceImpl
中添加实现
package com.stt.yygh.hosp.service.impl;
...
@Slf4j
@Service
public class HospitalServiceImpl implements HospitalService {
...
@Override
public String getHospName(String hoscode) {
Hospital hospital = repository.getHospitalByHoscode(hoscode);
if (Objects.isNull(hospital)) {
return "";
}
return hospital.getHosname();
}
}
2
3
4
5
6
7
8
9
10
11
12
13
14
15
# 排班日期分页列表前端实现
# 封装api
在 src/api/hosp.js中添加如下接口
// 查看排班列表
export function getScheduleRule(page, limit, hoscode, depcode) {
return request({
url: `/admin/hosp/schedule/getScheduleRule/${page}/${limit}/${hoscode}/${depcode}`,
method: 'get'
})
}
2
3
4
5
6
7
# 修改vue组件
修改/views/hosp/schedule.vue组件
<template>
<div class="app-container">
<div style="margin-bottom: 10px;font-size: 10px;">选择:{{ baseMap.hosname }} / {{ depname }} / {{ workDate }}</div>
<el-container style="height: 100%">
...
<el-main style="padding: 0 0 0 20px;">
<el-row style="width: 100%">
<!-- 排班日期 分页 -->
<el-tag v-for="(item,index) in bookingScheduleList" :key="item.id"
style="height: 60px;margin-right: 5px;margin-right:15px;cursor:pointer;"
:type="index == activeIndex ? '' : 'info'"
@click="selectDate(item.workDate, index)">
{{ item.workDate }} {{ item.dayOfWeek }}<br/>
{{ item.availableNumber }} / {{ item.reservedNumber }}
</el-tag>
<!-- 分页 -->
<el-pagination :current-page="page" :total="total" :page-size="limit" class="pagination"
layout="prev, pager, next"
@current-change="getPage" />
</el-row>
<!-- 排班日期对应的排班医生 -->
<el-row style="margin-top: 20px;"></el-row>
</el-main>
</el-container>
</div>
</template>
<script>
import { getDeptList, getScheduleRule } from '@/api/hosp'
export default {
data() {
return {
data: [],
defaultProps: {
children: 'children',
label: 'depname'
},
hoscode: null,
activeIndex: 0,
depcode: null,
depname: null,
workDate: null,
bookingScheduleList: [],
baseMap: {},
page: 1, // 当前页
limit: 7, // 每页个数
total: 0 // 总页码
}
},
created() {
this.hoscode = this.$route.params.hoscode
this.workDate = getCurDate()
this.fetchData()
function getCurDate() {
var datetime = new Date()
var year = datetime.getFullYear()
var month = datetime.getMonth() + 1 < 10 ? '0' + (datetime.getMonth() + 1) : datetime.getMonth() + 1
var date = datetime.getDate() < 10 ? '0' + datetime.getDate() : datetime.getDate()
return year + '-' + month + '-' + date
}
},
methods: {
fetchData(deptList = getDeptList) {
deptList(this.hoscode)
.then(response => {
this.data = response.data
// 默认选中第一个
if (this.data.length > 0) {
this.depcode = this.data[0].children[0].depcode
this.depname = this.data[0].children[0].depname
this.getPage()
}
})
},
getPage(page = 1) {
this.page = page
this.workDate = null
this.activeIndex = 0
this.getScheduleRule()
},
handleNodeClick(data) {
if (data.children != null) return
// 小科室才进行查询
this.depcode = data.depcode
this.depname = data.depname
this.getPage(1)
},
selectDate(workDate, index) {
this.workDate = workDate
this.activeIndex = index
},
getScheduleRule() {
getScheduleRule(this.page, this.limit, this.hoscode, this.depcode).then(res => {
this.bookingScheduleList = res.data.bookingScheduleRuleList
this.total = res.data.total
this.scheduleList = res.data.scheduleList
this.baseMap = res.data.baseMap
// 分页后workDate=null,默认选中第一个
if (this.workDate == null) {
this.workDate = this.bookingScheduleList[0].workDate
}
})
}
}
}
</script>
...
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
# 排班详情列表后端实现
# 添加controller
在service-hosp
创建ScheduleController
并添加接口方法
package com.stt.yygh.hosp.controller;
...
@RestController
@RequestMapping("/admin/hosp/schedule")
@CrossOrigin
public class ScheduleController {
@Autowired
private ScheduleService service;
...
//根据医院编号 、科室编号和工作日期,查询排班详细信息
@ApiOperation(value = "查询排班详细信息")
@GetMapping("detail/{hoscode}/{depcode}/{workDate}")
public Result getScheduleDetail( @PathVariable String hoscode,
@PathVariable String depcode,
@PathVariable String workDate) {
List<Schedule> list = service.getDetailSchedule(hoscode,depcode,workDate);
return Result.ok(list);
}
}
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
# 添加service
在service-hosp
添加ScheduleService
方法
package com.stt.yygh.hosp.service;
import com.stt.yygh.model.hosp.Schedule;
import com.stt.yygh.vo.hosp.ScheduleQueryVo;
import org.springframework.data.domain.Page;
import java.util.List;
import java.util.Map;
public interface ScheduleService {
void save(Map<String, Object> paramMap);
Page<Schedule> selectPage(int page, int limit, ScheduleQueryVo scheduleQueryVo);
void remove(String hoscode, String hosScheduleId);
Map<String, Object> getRuleSchedule(long page, long limit, String hoscode, String depcode);
List<Schedule> getDetailSchedule(String hoscode, String depcode, String workDate);
}
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
在service-hosp
添加ScheduleService
方法
package com.stt.yygh.hosp.service.impl;
...
@Service
public class ScheduleServiceImpl implements ScheduleService {
@Autowired
private ScheduleRepository repository;
@Autowired
private MongoTemplate mongoTemplate;
@Autowired
private HospitalService hospitalService;
@Autowired
private DepartmentService departmentService;
...
@Override
public List<Schedule> getDetailSchedule(String hoscode, String depcode, String workDate) {
List<Schedule> scheduleList = repository.findScheduleByHoscodeAndDepcodeAndWorkDate(hoscode, depcode,
new DateTime(workDate).toDate());
scheduleList.stream().forEach(s -> this.packageSchedule(s));
return scheduleList;
}
// 设置其他值:医院名称、科室名称、日期对应星期
private Schedule packageSchedule(Schedule s) {
//设置医院名称
s.getParam().put("hosname",hospitalService.getHospName(s.getHoscode()));
//设置科室名称
s.getParam().put("depname",departmentService.getDepName(s.getHoscode(),s.getDepcode()));
return s;
}
}
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
# 添加根据部门编码获取部门名称
在DepartmentService类添加接口
package com.stt.yygh.hosp.service;
...
public interface DepartmentService {
...
String getDepName(String hoscode, String depcode);
}
2
3
4
5
6
在DepartmentServiceImpl类添加接口实现
package com.stt.yygh.hosp.service.impl;
...
@Service
public class DepartmentServiceImpl implements DepartmentService {
@Autowired
private DepartmentRepository repository;
...
@Override
public String getDepName(String hoscode, String depcode) {
Department department = repository.getDepartmentByHoscodeAndDepcode(hoscode, depcode);
if(Objects.isNull(department)) {
return "";
}
return department.getDepname();
}
}
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
# 排班详情列表前端实现
# 封装api
在 src/api/hosp.js中添加如下接口
// 查询排班详情
export function getScheduleDetail(hoscode, depcode, workDate) {
return request({
url: `/admin/hosp/schedule/detail/${hoscode}/${depcode}/${workDate}`,
method: 'get'
})
}
2
3
4
5
6
7
# 修改vue组件
修改/views/hosp/schedule.vue组件
<template>
<div class="app-container">
...
<el-container style="height: 100%">
<!-- 部门 -->
<el-aside width="200px" style="border: 1px silver solid">
...
</el-aside>
<el-main style="padding: 0 0 0 20px;">
<!-- 排班日期 分页 -->
<el-row style="width: 100%">
...
</el-row>
<!-- 排班日期对应的排班医生 -->
<el-row style="margin-top: 20px;">
<el-table v-loading="listLoading" :data="scheduleList" border fit highlight-current-row>
<el-table-column label="序号" width="60" align="center">
<template slot-scope="scope">{{ scope.$index + 1 }}</template>
</el-table-column>
<el-table-column label="职称" width="150">
<template slot-scope="scope">{{ scope.row.title }} | {{ scope.row.docname }}</template>
</el-table-column>
<el-table-column label="号源时间" width="80">
<template slot-scope="scope">{{ scope.row.workTime == 0 ? '上午' : '下午' }}</template>
</el-table-column>
<el-table-column prop="reservedNumber" label="可预约数" width="80"/>
<el-table-column prop="availableNumber" label="剩余预约数" width="100"/>
<el-table-column prop="amount" label="挂号费(元)" width="90"/>
<el-table-column prop="skill" label="擅长技能"/>
</el-table>
</el-row>
</el-main>
</el-container>
</div>
</template>
<script>
import { getDeptList, getScheduleDetail, getScheduleRule } from '@/api/hosp'
export default {
data() {
return {
...
listLoading: false,
scheduleList: [] // 排班详情
}
},
created() {
...
},
methods: {
...
selectDate(workDate, index) {
this.workDate = workDate
this.activeIndex = index
// 调用查询排班详情
this.getDetailSchedule()
},
getScheduleRule() {
getScheduleRule(this.page, this.limit, this.hoscode, this.depcode).then(res => {
this.bookingScheduleList = res.data.bookingScheduleRuleList
this.total = res.data.total
this.scheduleList = res.data.scheduleList
this.baseMap = res.data.baseMap
// 分页后workDate=null,默认选中第一个
if (this.workDate == null) {
this.workDate = this.bookingScheduleList[0].workDate
}
// 调用查询排班详情
this.getDetailSchedule()
})
},
// 查询排班详情
getDetailSchedule() {
getScheduleDetail(this.hoscode, this.depcode, this.workDate)
.then(res => {
this.scheduleList = res.data
})
}
}
}
</script>
...
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82