29.前台系统-预约挂号详情
# 需求分析
从平台首页点击某个医院,进入医院详情后,点击科室和门诊进入到【预约挂号详情页面】
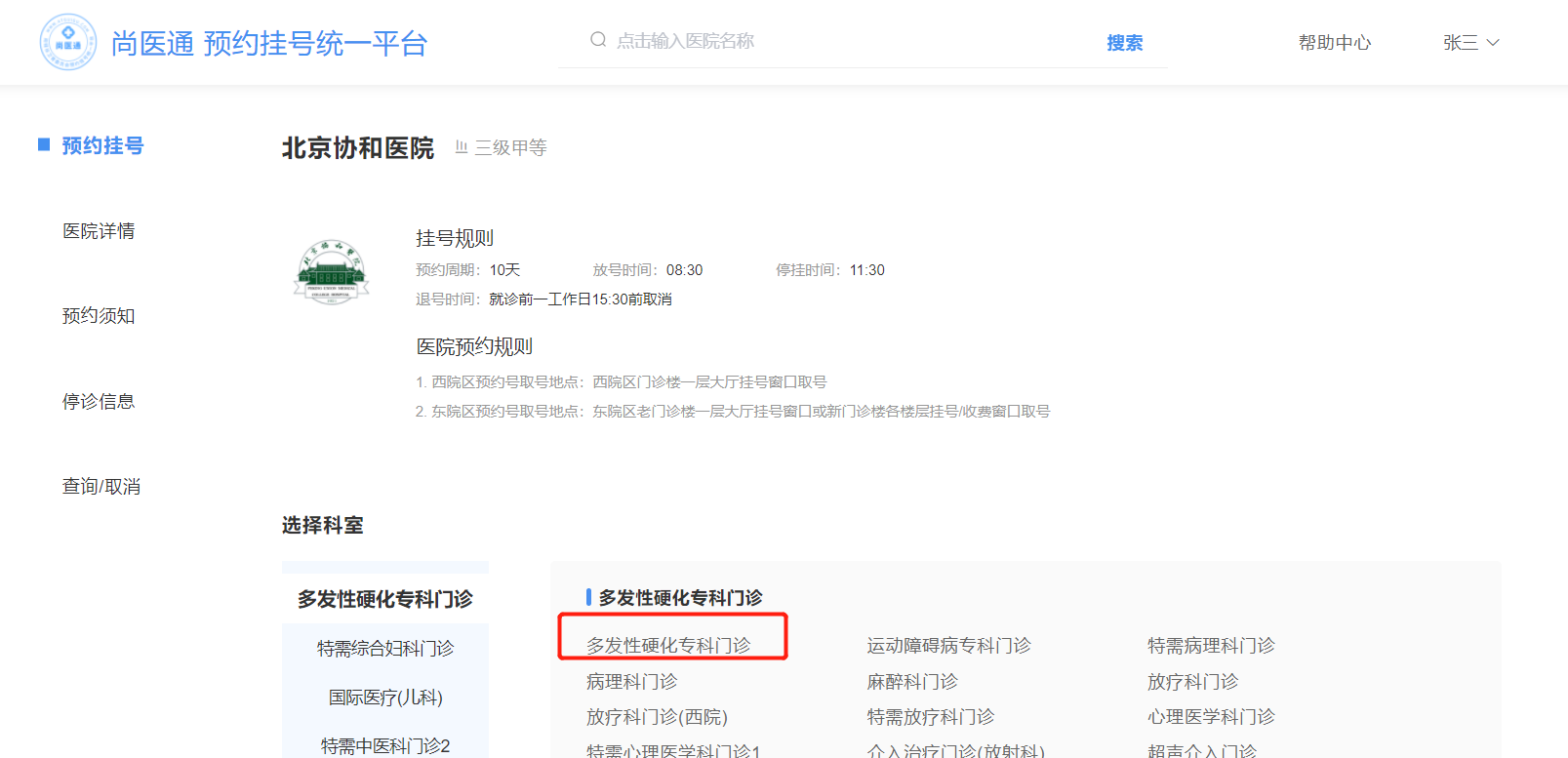
接口分析
- 根据预约周期,展示可预约日期数据,按分页展示
- 选择日期展示当天可预约列表(该接口后台已经实现过)
页面展示分析
- 分页展示可预约日期,根据有号、无号、约满等状态展示不同颜色,以示区分
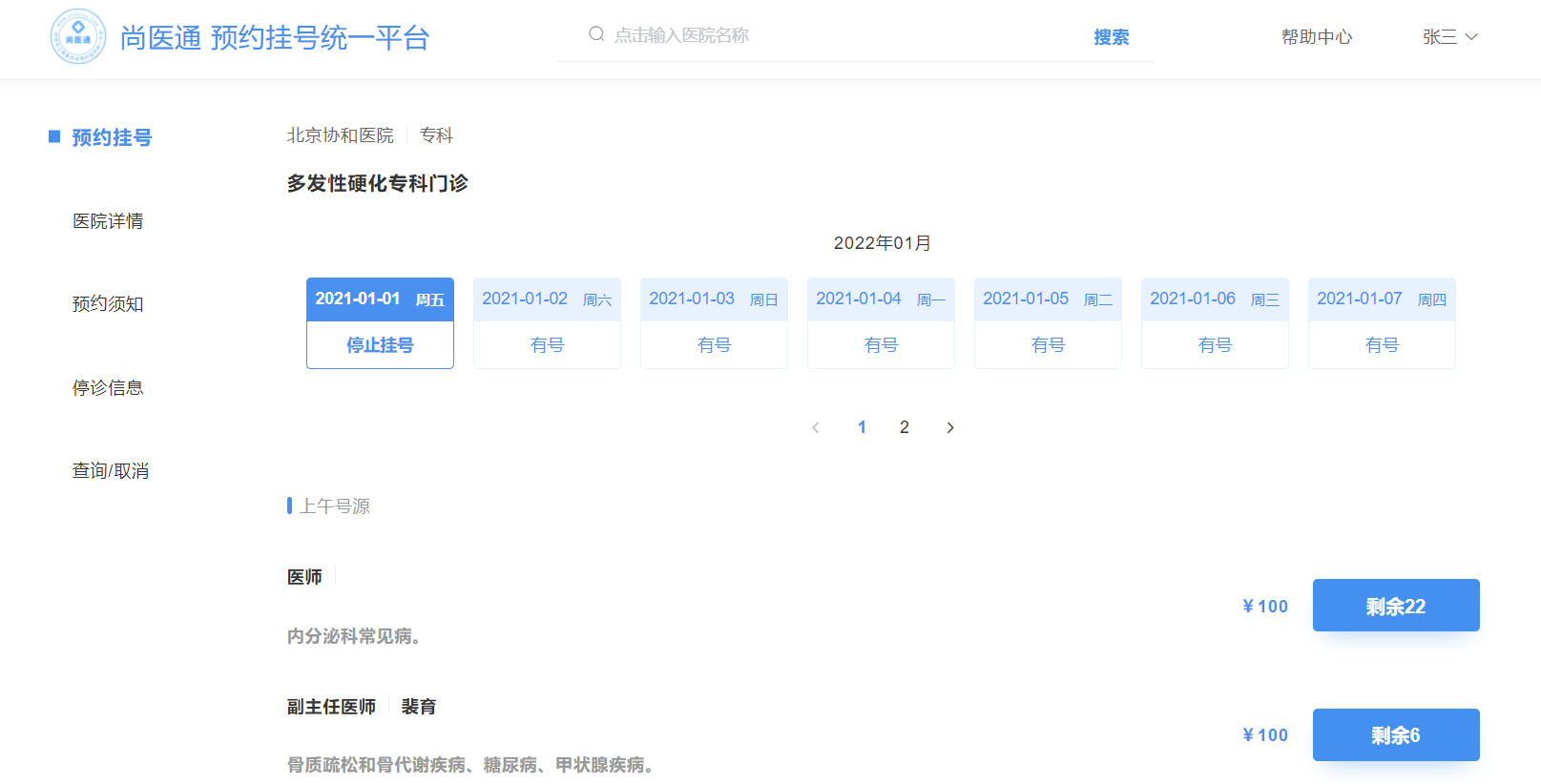
- 可预约最后一个日期为即将放号日期,根据放号时间页面展示倒计时
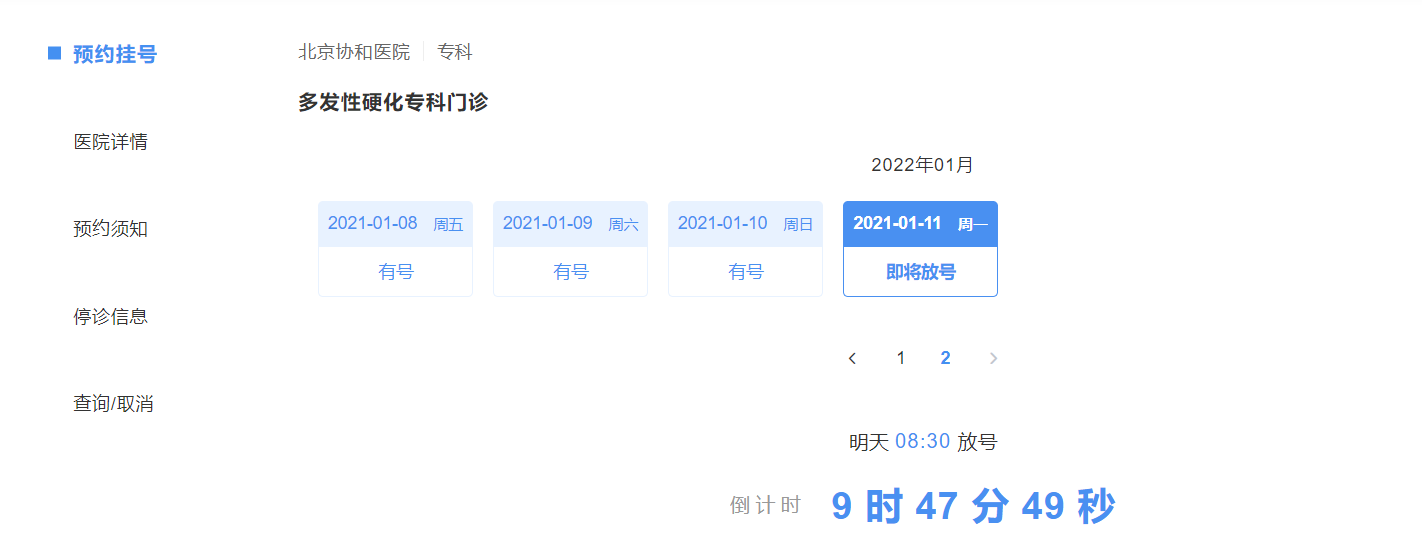
# 后端实现 service-hosp
# 添加service接口与实现
# 获取预约挂号明细
在 ScheduleService
类添加接口
package com.stt.yygh.hosp.service;
import com.stt.yygh.model.hosp.Schedule;
import com.stt.yygh.vo.hosp.ScheduleQueryVo;
import org.springframework.data.domain.Page;
import java.util.List;
import java.util.Map;
public interface ScheduleService {
...
/**
* 获取排班可预约日期数据
* @param page
* @param limit
* @param hoscode
* @param depcode
* @return
*/
Map<String, Object> getBookingScheduleRule(int page, int limit, String hoscode, String depcode);
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
添加实现
- 先从预约规则中获取预约的日期列表
- 从医院的科室中进行聚合查询,通过日期列表得到可预约的日期和当前可用号个数以及医生个数等信息
- 注意:由于上传的科室的数据中,有挂号数据的日期从2021-01-01开始,因此在此处为了方便测试,添加测试语句,固定查询日期,后期实际使用需要去除
package com.stt.yygh.hosp.service.impl;
import com.alibaba.fastjson.JSONObject;
import com.baomidou.mybatisplus.core.metadata.IPage;
import com.stt.yygh.common.exception.YyghException;
import com.stt.yygh.common.result.ResultCodeEnum;
import com.stt.yygh.hosp.repository.ScheduleRepository;
import com.stt.yygh.hosp.service.DepartmentService;
import com.stt.yygh.hosp.service.HospitalService;
import com.stt.yygh.hosp.service.ScheduleService;
import com.stt.yygh.model.hosp.BookingRule;
import com.stt.yygh.model.hosp.Department;
import com.stt.yygh.model.hosp.Hospital;
import com.stt.yygh.model.hosp.Schedule;
import com.stt.yygh.vo.hosp.BookingScheduleRuleVo;
import com.stt.yygh.vo.hosp.ScheduleQueryVo;
import org.joda.time.DateTime;
import org.joda.time.DateTimeConstants;
import org.joda.time.format.DateTimeFormat;
import org.springframework.beans.BeanUtils;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.domain.*;
import org.springframework.data.mongodb.core.MongoTemplate;
import org.springframework.data.mongodb.core.aggregation.Aggregation;
import org.springframework.data.mongodb.core.aggregation.AggregationResults;
import org.springframework.data.mongodb.core.query.Criteria;
import org.springframework.stereotype.Service;
import java.util.*;
import java.util.stream.Collectors;
@Service
public class ScheduleServiceImpl implements ScheduleService {
@Autowired
private ScheduleRepository repository;
@Autowired
private MongoTemplate mongoTemplate;
@Autowired
private HospitalService hospitalService;
@Autowired
private DepartmentService departmentService;
...
@Override
public Map<String, Object> getBookingScheduleRule(int page, int limit, String hoscode, String depcode) {
//获取预约规则
Hospital hospital = hospitalService.getByHoscode(hoscode);
if (Objects.isNull(hospital)) throw new YyghException(ResultCodeEnum.DATA_ERROR);
BookingRule bookingRule = hospital.getBookingRule();
//获取可预约日期分页数据
IPage<Date> datePage = this.getListDate(page, limit, bookingRule);
//获取可预约日期科室剩余预约数
Aggregation agg = Aggregation.newAggregation(
Aggregation.match(Criteria
.where("hoscode").is(hoscode)
.and("depcode").is(depcode)
.and("workDate").in(datePage.getRecords())), //当前页可预约日期
Aggregation.group("workDate")//分组字段
.first("workDate").as("workDate")
.count().as("docCount")
.sum("availableNumber").as("availableNumber")
.sum("reservedNumber").as("reservedNumber")
);
//获取科室剩余预约数
List<BookingScheduleRuleVo> scheduleVoList = mongoTemplate.aggregate(agg, Schedule.class, BookingScheduleRuleVo.class)
.getMappedResults();
//合并数据 将统计数据ScheduleVo根据“安排日期”合并到BookingRuleVo
Map<Date, BookingScheduleRuleVo> scheduleVoMap = scheduleVoList.stream()
.collect(Collectors.toMap(BookingScheduleRuleVo::getWorkDate, v -> v));
//获取可预约排班规则
List<BookingScheduleRuleVo> bookingScheduleRuleVoList = new ArrayList<>();
for (int i = 0, len = datePage.getRecords().size(); i < len; i++) {
Date date = datePage.getRecords().get(i);
BookingScheduleRuleVo rule = scheduleVoMap.get(date);
if (Objects.isNull(rule)) { // 说明当天没有排班医生
rule = new BookingScheduleRuleVo();
rule.setDocCount(0); //就诊医生人数
rule.setAvailableNumber(-1); //科室剩余预约数 -1表示无号
}
rule.setWorkDate(date);
rule.setWorkDateMd(date);
rule.setDayOfWeek(this.getDayOfWeek(new DateTime(date))); //计算当前预约日期为周几
//最后一页最后一条记录为即将预约 状态 0:正常 1:即将放号 -1:当天已停止挂号
if (i == len - 1 && page == datePage.getPages()) {
rule.setStatus(1);
} else {
rule.setStatus(0);
}
//当天预约如果过了停号时间, 不能预约
if (i == 0 && page == 1) {
DateTime stopTime = this.getDateTime(new Date(), bookingRule.getStopTime());
if (stopTime.isBeforeNow()) rule.setStatus(-1); //停止预约
}
bookingScheduleRuleVoList.add(rule);
}
Map<String, Object> re = new HashMap<>();
//可预约日期规则数据
re.put("bookingScheduleList", bookingScheduleRuleVoList);
re.put("total", datePage.getTotal());
//其他基础数据
Map<String, String> baseMap = new HashMap<>();
//医院名称
baseMap.put("hosname", hospitalService.getHospName(hoscode));
//科室
Department department = departmentService.getDepartment(hoscode, depcode);
baseMap.put("bigname", department.getBigname()); //大科室名称
baseMap.put("depname", department.getDepname()); //科室名称
baseMap.put("workDateString", new DateTime().toString("yyyy年MM月")); //月
baseMap.put("releaseTime", bookingRule.getReleaseTime()); //放号时间
baseMap.put("stopTime", bookingRule.getStopTime()); //停号时间
re.put("baseMap", baseMap);
return re;
}
/**
* 获取可预约日期分页数据
*/
private IPage<Date> getListDate(int page, int limit, BookingRule bookingRule) {
// 由于测试数据的预约挂号时间是2021-03-02开始,因此可以设置固定时间用于前端数据显示,供测试使用,后期测试完成后需要删除
DateTime testDate = DateTimeFormat.forPattern("yyyy-MM-dd").parseDateTime("2021-01-01");
DateTime releaseTime = this.getDateTime(testDate.toDate(), bookingRule.getReleaseTime());
//当天放号时间
// DateTime releaseTime = this.getDateTime(new Date(), bookingRule.getReleaseTime());
//预约周期
int cycle = bookingRule.getCycle();
//如果当天放号时间已过,则预约周期后一天为即将放号时间,周期加1
if (releaseTime.isBeforeNow()) cycle += 1;
//可预约所有日期,最后一天显示即将放号倒计时
List<Date> dateList = new ArrayList<>();
for (int i = 0; i < cycle; i++) {
// 使用如下语句只是用于测试使用,后期需要删除
DateTime curDateTime = testDate.plusDays(i);
//计算当前预约日期
// DateTime curDateTime = new DateTime().plusDays(i);
String dateString = curDateTime.toString("yyyy-MM-dd");
dateList.add(new DateTime(dateString).toDate());
}
//日期分页,由于预约周期不一样,页面一排最多显示7天数据,多了就要分页显示
List<Date> pageDateList = new ArrayList<>();
int start = (page - 1) * limit;
int end = (page - 1) * limit + limit;
if (end > dateList.size()) end = dateList.size();
for (int i = start; i < end; i++) {
pageDateList.add(dateList.get(i));
}
IPage<Date> re = new com.baomidou.mybatisplus.extension.plugins.pagination.Page(page, 7, dateList.size());
re.setRecords(pageDateList);
return re;
}
/**
* 将Date日期(yyyy-MM-dd HH:mm)转换为DateTime
*/
private DateTime getDateTime(Date date, String timeString) {
String dateTimeString = new DateTime(date).toString("yyyy-MM-dd") + " " + timeString;
return DateTimeFormat.forPattern("yyyy-MM-dd HH:mm").parseDateTime(dateTimeString);
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
134
135
136
137
138
139
140
141
142
143
144
145
146
147
148
149
150
151
152
153
154
155
156
157
158
159
160
161
162
163
164
165
166
167
168
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
134
135
136
137
138
139
140
141
142
143
144
145
146
147
148
149
150
151
152
153
154
155
156
157
158
159
160
161
162
163
164
165
166
167
168
# 获取科室信息
在DepartmentService
中添加接口和实现
package com.stt.yygh.hosp.service;
import com.stt.yygh.model.hosp.Department;
import com.stt.yygh.vo.hosp.DepartmentQueryVo;
import com.stt.yygh.vo.hosp.DepartmentVo;
import org.springframework.data.domain.Page;
import java.util.List;
import java.util.Map;
public interface DepartmentService {
...
Department getDepartment(String hoscode, String depcode);
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
2
3
4
5
6
7
8
9
10
11
12
13
14
在DepartmentServiceImpl
中的实现
package com.stt.yygh.hosp.service.impl;
import com.alibaba.fastjson.JSONObject;
import com.stt.yygh.hosp.repository.DepartmentRepository;
import com.stt.yygh.hosp.service.DepartmentService;
import com.stt.yygh.model.hosp.Department;
import com.stt.yygh.vo.hosp.DepartmentQueryVo;
import com.stt.yygh.vo.hosp.DepartmentVo;
import org.springframework.beans.BeanUtils;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.domain.*;
import org.springframework.stereotype.Service;
import java.util.*;
import java.util.stream.Collectors;
@Service
public class DepartmentServiceImpl implements DepartmentService {
...
@Override
public Department getDepartment(String hoscode, String depcode) {
return repository.getDepartmentByHoscodeAndDepcode(hoscode, depcode);
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
# 添加controller接口
在 HospitalApiController
中添加接口
package com.stt.yygh.hosp.controller;
import com.stt.yygh.common.result.Result;
import com.stt.yygh.hosp.service.DepartmentService;
import com.stt.yygh.hosp.service.HospitalService;
import com.stt.yygh.hosp.service.ScheduleService;
import com.stt.yygh.model.hosp.Hospital;
import com.stt.yygh.vo.hosp.HospitalQueryVo;
import io.swagger.annotations.Api;
import io.swagger.annotations.ApiOperation;
import io.swagger.annotations.ApiParam;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.domain.Page;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import java.util.List;
@Api(tags = "医院管理接口")
@RestController
@RequestMapping("/api/hosp/hospital")
public class HospitalApiController {
...
@Autowired
private ScheduleService scheduleService;
...
@ApiOperation(value = "获取可预约排班数据")
@GetMapping("auth/getBookingScheduleRule/{page}/{limit}/{hoscode}/{depcode}")
public Result getBookingSchedule(@PathVariable Integer page,
@PathVariable Integer limit,
@PathVariable String hoscode,
@PathVariable String depcode) {
return Result.ok(scheduleService.getBookingScheduleRule(page, limit, hoscode, depcode));
}
// workDate 排班日期
// depcode 科室code
// hoscode 医院code
@ApiOperation(value = "获取排班数据")
@GetMapping("auth/findScheduleList/{hoscode}/{depcode}/{workDate}")
public Result findScheduleList(@PathVariable String hoscode,
@PathVariable String depcode,
@PathVariable String workDate) {
return Result.ok(scheduleService.getDetailSchedule(hoscode, depcode, workDate));
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
# 前端实现 yygh-site
# 封装api
在/api/hosp/hosp.js添加方法
import request from '~/utils/request'
const api_name = `/api/hosp/hospital`
...
export function getBookingScheduleRule(page, limit, hoscode, depcode) {
return request({
url: `${api_name}/auth/getBookingScheduleRule/${page}/${limit}/${hoscode}/${depcode}`,
method: 'get'
})
}
export function findScheduleList(hoscode, depcode, workDate) {
return request({
url: `${api_name}/auth/findScheduleList/${hoscode}/${depcode}/${workDate}`,
method: 'get'
})
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
# 创建预约挂号页面
由于该页面是通过科室点击进入,而科室点击按键(在pages/hospital/_hoscode.vue
页面)会的跳转逻辑如下
schedule(depcode) {
// 登录判断
let token = cookie.get('token')
if (!token) {
loginEvent.$emit('loginDialogEvent')
return
}
//判断认证
getUserInfo().then(res => {
let authStatus = res.data.authStatus
// 状态为2认证通过
if (!authStatus || authStatus != 2) {
window.location.href = '/user'
return
}
window.location.href = '/hospital/schedule?hoscode=' + this.hospital.hoscode + '&depcode=' + depcode
})
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
从跳转的路径中可以获取到hoscode和depcode信息传递给hospital/schedule.vue
页面
创建/pages/hospital/schedule.vue
组件
<template>
<!-- header -->
<div class="nav-container page-component">
<!--左侧导航 #start -->
<div class="nav left-nav">
<div class="nav-item selected">
<span class="v-link selected dark" :onclick="'javascript:window.location=\'/hosp/'+hoscode+'\''">预约挂号 </span>
</div>
<div class="nav-item ">
<span class="v-link clickable dark"
:onclick="'javascript:window.location=\'/hosp/detail/'+hoscode+'\''"> 医院详情 </span>
</div>
<div class="nav-item">
<span class="v-link clickable dark"
:onclick="'javascript:window.location=\'/hosp/notice/'+hoscode+'\''"> 预约须知 </span>
</div>
<div class="nav-item "><span class="v-link clickable dark">停诊信息</span></div>
<div class="nav-item "><span class="v-link clickable dark">查询/取消</span></div>
</div>
<!-- 左侧导航 #end -->
<!-- 右侧内容 #start -->
<div class="page-container">
<div class="hospital-source-list">
<div class="header-wrapper" style="justify-content:normal">
<span class="v-link clickable" @click="show()">{{ baseMap.hosname }}</span>
<div class="split"></div>
<div>{{ baseMap.bigname }}</div>
</div>
<div class="title mt20"> {{ baseMap.depname }}</div>
<!-- 号源列表 #start -->
<div class="mt60">
<div class="title-wrapper">{{ baseMap.workDateString }}</div>
<div class="calendar-list-wrapper">
<div :class="'calendar-item '+item.curClass" style="width: 124px;"
v-for="(item, index) in bookingScheduleList" :key="item.id"
@click="selectDate(item, index)">
<div class="date-wrapper"><span>{{ item.workDate }}</span><span class="week">{{ item.dayOfWeek }}</span>
</div>
<div class="status-wrapper" v-if="item.status == 0">
{{ item.availableNumber == -1 ? '无号' : item.availableNumber == 0 ? '约满' : '有号' }}
</div>
<div class="status-wrapper" v-if="item.status == 1">即将放号</div>
<div class="status-wrapper" v-if="item.status == -1">停止挂号</div>
</div>
</div>
<!-- 分页 -->
<el-pagination class="pagination" layout="prev, pager, next" :current-page="page"
:total="total" :page-size="limit" @current-change="getPage"/>
</div>
<!-- 即将放号 #start-->
<div class="countdown-wrapper mt60" v-if="!tabShow">
<div class="countdonw-title">{{ time }}<span class="v-link selected">{{ baseMap.releaseTime }}</span>放号</div>
<div class="countdown-text">倒计时<span class="number">{{ timeString }}</span></div>
</div>
<!-- 即将放号 #end-->
<!-- 号源列表 #end -->
<!-- 上午号源 #start -->
<div class="mt60" v-if="tabShow">
<div class="">
<div class="list-title"><span class="block"></span>上午号源</div>
<div v-for="item in scheduleList" :key="item.id" v-if="item.workTime == 0">
<div class="list-item">
<div class="item-wrapper">
<div class="title-wrapper">
<div class="title">{{ item.title }}</div>
<div class="split"></div>
<div class="name"> {{ item.docname }}</div>
</div>
<div class="special-wrapper">{{ item.skill }}</div>
</div>
<div class="right-wrapper">
<div class="fee"> ¥{{ item.amount }}</div>
<div class="button-wrapper">
<div class="v-button" @click="booking(item.id, item.availableNumber)"
:style="item.availableNumber == 0 || pageFirstStatus == -1 ? 'background-color: #7f828b;' : ''">
<span>剩余<span class="number">{{ item.availableNumber }}</span></span></div>
</div>
</div>
</div>
</div>
</div>
</div>
<!-- 上午号源 #end -->
<!-- 下午号源 #start -->
<div class="mt60" v-if="tabShow">
<div class="">
<div class="list-title"><span class="block"></span>下午号源</div>
<div v-for="item in scheduleList" :key="item.id" v-if="item.workTime == 1">
<div class="list-item">
<div class="item-wrapper">
<div class="title-wrapper">
<div class="title">{{ item.title }}</div>
<div class="split"></div>
<div class="name">{{ item.docname }}</div>
</div>
<div class="special-wrapper">{{ item.skill }}</div>
</div>
<div class="right-wrapper">
<div class="fee">¥{{ item.amount }}</div>
<div class="button-wrapper">
<div class="v-button" @click="booking(item.id, item.availableNumber)"
:style="item.availableNumber == 0 || pageFirstStatus == -1 ? 'background-color: #7f828b;' : ''">
<span>剩余<span class="number">{{ item.availableNumber }}</span></span></div>
</div>
</div>
</div>
</div>
</div>
</div>
<!-- 下午号源 #end -->
</div>
</div>
<!-- 右侧内容 #end -->
</div>
<!-- footer -->
</template>
<script>
import '~/assets/css/hospital_personal.css'
import '~/assets/css/hospital.css'
import { findScheduleList, getBookingScheduleRule } from '@/api/hosp/hosp'
export default {
data() {
return {
hoscode: null,
depcode: null,
workDate: null,
bookingScheduleList: [],
scheduleList: [],
baseMap: {},
nextWorkDate: null, // 下一页第一个日期
preWorkDate: null, // 上一页第一个日期
tabShow: true, //挂号列表与即将挂号切换
activeIndex: 0,
page: 1, // 当前页
limit: 7, // 每页个数
total: 1, // 总页码
timeString: null,
time: '今天',
timer: null,
pageFirstStatus: 0 // 第一页第一条数据状态
}
},
created() {
this.hoscode = this.$route.query.hoscode
this.depcode = this.$route.query.depcode
this.workDate = this.getCurDate()
this.getBookingScheduleRule()
},
methods: {
getPage(page = 1) {
this.page = page
this.workDate = null
this.activeIndex = 0
this.getBookingScheduleRule()
},
getBookingScheduleRule() {
getBookingScheduleRule(this.page, this.limit, this.hoscode, this.depcode).then(res => {
this.bookingScheduleList = res.data.bookingScheduleList
this.total = res.data.total
this.baseMap = res.data.baseMap
this.dealClass()
// 分页后workDate=null,默认选中第一个
if (this.workDate == null) this.workDate = this.bookingScheduleList[0].workDate
//判断当天是否停止预约 status == -1 停止预约
if (this.workDate === this.getCurDate()) {
this.pageFirstStatus = this.bookingScheduleList[0].status
} else {
this.pageFirstStatus = 0
}
this.findScheduleList()
})
},
findScheduleList() {
findScheduleList(this.hoscode, this.depcode, this.workDate).then(res => {
this.scheduleList = res.data
})
},
selectDate(item, index) {
this.workDate = item.workDate
this.activeIndex = index
//清理定时
clearInterval(this.timer)
// 是否即将放号
if (item.status == 1) {
this.tabShow = false
// 放号时间
let releaseTime = new Date(this.getCurDate() + ' ' + this.baseMap.releaseTime).getTime()
let nowTime = new Date().getTime()
this.countDown(releaseTime, nowTime)
this.dealClass()
} else {
this.tabShow = true
this.getBookingScheduleRule()
}
},
dealClass() {
//处理样式
for (let i = 0; i < this.bookingScheduleList.length; i++) {
// depNumber -1:无号 0:约满 >0:有号
let curClass = this.bookingScheduleList[i].availableNumber == -1 ? 'gray space' : this.bookingScheduleList[i].availableNumber == 0 ? 'gray' : 'small small-space'
curClass += i == this.activeIndex ? ' selected' : ''
this.bookingScheduleList[i].curClass = curClass
}
},
getCurDate() {
let datetime = new Date()
let year = datetime.getFullYear()
let month = datetime.getMonth() + 1 < 10 ? '0' + (datetime.getMonth() + 1) : datetime.getMonth() + 1
let date = datetime.getDate() < 10 ? '0' + datetime.getDate() : datetime.getDate()
return year + '-' + month + '-' + date
},
countDown(releaseTime, nowTime) {
//计算倒计时时长
let secondes = 0
if (releaseTime > nowTime) {
this.time = '今天'
//当前时间到放号时间的时长
secondes = Math.floor((releaseTime - nowTime) / 1000)
} else {
this.time = '明天'
//计算明天放号时间
let releaseDate = new Date(releaseTime)
releaseTime = new Date(releaseDate.setDate(releaseDate.getDate() + 1)).getTime()
//当前时间到明天放号时间的时长
secondes = Math.floor((releaseTime - nowTime) / 1000)
}
//定时任务
this.timer = setInterval(() => {
secondes = secondes - 1
if (secondes <= 0) {
clearInterval(timer)
this.init()
}
this.timeString = this.convertTimeString(secondes)
}, 1000)
// 通过$once来监听定时器,在beforeDestroy钩子可以被清除。
this.$once('hook:beforeDestroy', () => {
clearInterval(timer)
})
},
convertTimeString(allseconds) {
if (allseconds <= 0) return '00:00:00'
let days = Math.floor(allseconds / (60 * 60 * 24)) // 计算天数
let hours = Math.floor((allseconds - (days * 60 * 60 * 24)) / (60 * 60)) // 小时
let minutes = Math.floor((allseconds - (days * 60 * 60 * 24) - (hours * 60 * 60)) / 60) // 分钟
let seconds = allseconds - (days * 60 * 60 * 24) - (hours * 60 * 60) - (minutes * 60) // 秒
//拼接时间
let timString = ''
if (days > 0) timString = days + '天:'
return timString += hours + ' 时 ' + minutes + ' 分 ' + seconds + ' 秒 '
},
show() {
window.location.href = '/hospital/' + this.hoscode
},
booking(scheduleId, availableNumber) {
if (availableNumber == 0 || this.pageFirstStatus == -1) {
this.$message.error('不能预约')
} else {
window.location.href = '/hospital/booking?scheduleId=' + scheduleId
}
}
}
}
</script>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
134
135
136
137
138
139
140
141
142
143
144
145
146
147
148
149
150
151
152
153
154
155
156
157
158
159
160
161
162
163
164
165
166
167
168
169
170
171
172
173
174
175
176
177
178
179
180
181
182
183
184
185
186
187
188
189
190
191
192
193
194
195
196
197
198
199
200
201
202
203
204
205
206
207
208
209
210
211
212
213
214
215
216
217
218
219
220
221
222
223
224
225
226
227
228
229
230
231
232
233
234
235
236
237
238
239
240
241
242
243
244
245
246
247
248
249
250
251
252
253
254
255
256
257
258
259
260
261
262
263
264
265
266
267
268
269
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
134
135
136
137
138
139
140
141
142
143
144
145
146
147
148
149
150
151
152
153
154
155
156
157
158
159
160
161
162
163
164
165
166
167
168
169
170
171
172
173
174
175
176
177
178
179
180
181
182
183
184
185
186
187
188
189
190
191
192
193
194
195
196
197
198
199
200
201
202
203
204
205
206
207
208
209
210
211
212
213
214
215
216
217
218
219
220
221
222
223
224
225
226
227
228
229
230
231
232
233
234
235
236
237
238
239
240
241
242
243
244
245
246
247
248
249
250
251
252
253
254
255
256
257
258
259
260
261
262
263
264
265
266
267
268
269
Last Updated: 2022/01/16, 11:29:51