28.后台系统-平台用户管理
# 管理后台-平台用户管理
前面做了用户登录、用户认证与就诊人,现在需要把这些信息在后台管理系统做统一管理
操作模块:service-user
实现的功能
- 用户列表
- 锁定
- 详情
- 用户认证列表
- 用户认证审批
# 页面效果
- 用户列表页,包含查看详情,锁定按键
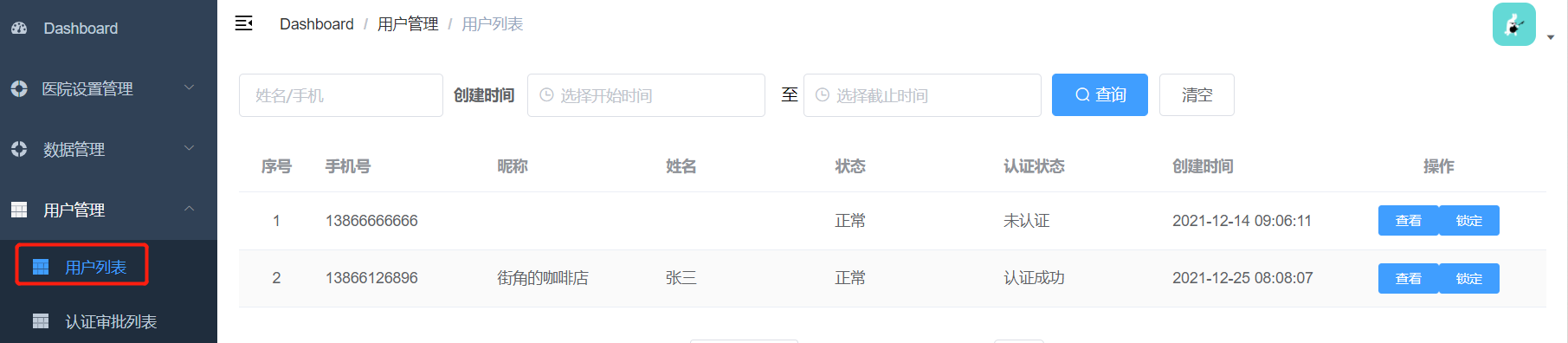
- 用户详情页
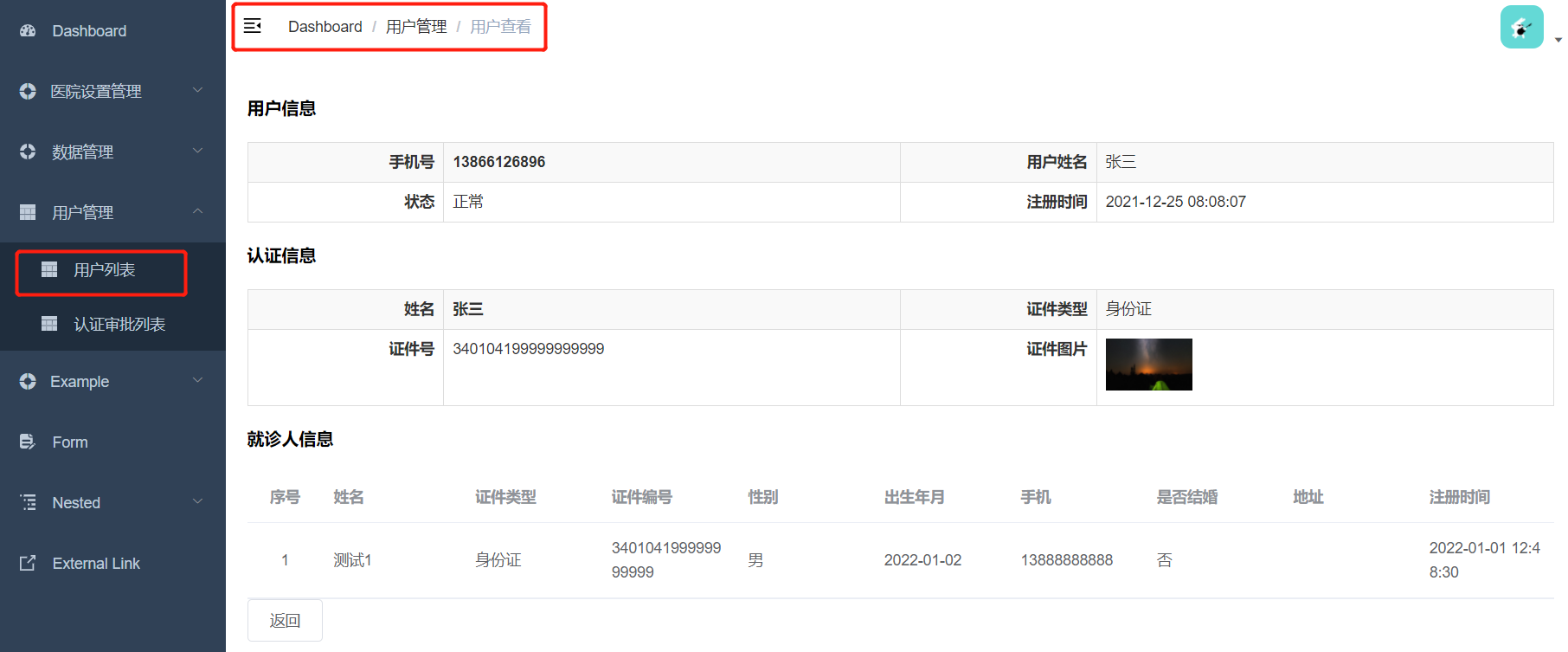
- 认证审核列表,与用户列表类似,查看详情也是一致的
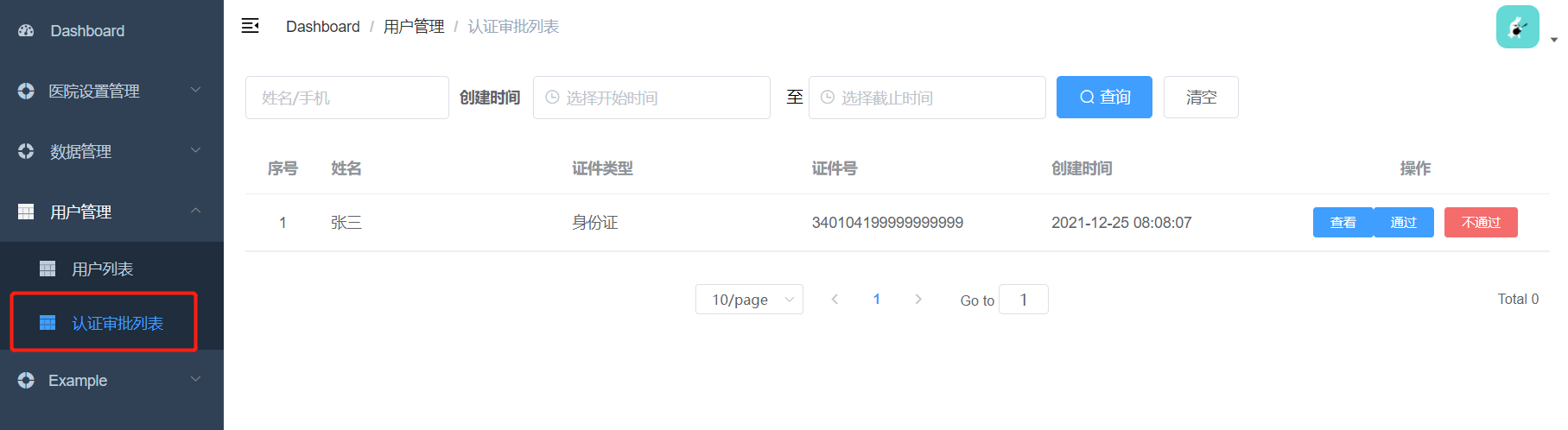
# 用户列表 service-user
# 创建查询vo类
在 module
模块中创建com.stt.yygh.vo.user.UserInfoQueryVo
类
package com.stt.yygh.vo.user;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import lombok.Data;
@Data
@ApiModel(description="会员搜索对象")
public class UserInfoQueryVo {
@ApiModelProperty(value = "关键字")
private String keyword;
@ApiModelProperty(value = "状态")
private Integer status;
@ApiModelProperty(value = "认证状态")
private Integer authStatus;
@ApiModelProperty(value = "创建时间")
private String createTimeBegin;
@ApiModelProperty(value = "创建时间")
private String createTimeEnd;
}
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
# 添加service接口与实现
在service-user
模块中修改UserInfoService
,添加用户分页查询方法
package com.stt.yygh.user.service;
...
public interface UserInfoService extends IService<UserInfo> {
...
//用户列表(条件查询带分页)
Page<UserInfo> selectPage(Page<UserInfo> pageParam, UserInfoQueryVo userInfoQueryVo);
}
2
3
4
5
6
7
在UserInfoServiceImpl
类实现该分页方法
package com.stt.yygh.user.service.impl;
import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper;
import com.baomidou.mybatisplus.extension.plugins.pagination.Page;
import com.baomidou.mybatisplus.extension.service.impl.ServiceImpl;
import com.stt.yygh.common.exception.YyghException;
import com.stt.yygh.common.helper.JwtHelper;
import com.stt.yygh.common.result.ResultCodeEnum;
import com.stt.yygh.enums.AuthStatusEnum;
import com.stt.yygh.model.user.UserInfo;
import com.stt.yygh.user.mapper.UserInfoMapper;
import com.stt.yygh.user.service.UserInfoService;
import com.stt.yygh.vo.user.LoginVo;
import com.stt.yygh.vo.user.UserAuthVo;
import com.stt.yygh.vo.user.UserInfoQueryVo;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.redis.core.RedisTemplate;
import org.springframework.stereotype.Service;
import org.springframework.util.StringUtils;
import java.util.HashMap;
import java.util.Map;
import java.util.Objects;
@Service
public class UserInfoServiceImpl extends ServiceImpl<UserInfoMapper, UserInfo> implements UserInfoService {
...
@Override
public Page<UserInfo> selectPage(Page<UserInfo> pageParam, UserInfoQueryVo userInfoQueryVo) {
//UserInfoQueryVo获取条件值
String name = userInfoQueryVo.getKeyword(); //用户名称
Integer status = userInfoQueryVo.getStatus();//用户状态
Integer authStatus = userInfoQueryVo.getAuthStatus(); //认证状态
String createTimeBegin = userInfoQueryVo.getCreateTimeBegin(); //开始时间
String createTimeEnd = userInfoQueryVo.getCreateTimeEnd(); //结束时间
//对条件值进行非空判断
QueryWrapper<UserInfo> wrapper = new QueryWrapper<>();
if (!StringUtils.isEmpty(name)) {
wrapper.like("name", name);
}
if (!StringUtils.isEmpty(status)) {
wrapper.eq("status", status);
}
if (!StringUtils.isEmpty(authStatus)) {
wrapper.eq("auth_status", authStatus);
}
if (!StringUtils.isEmpty(createTimeBegin)) {
wrapper.ge("create_time", createTimeBegin);
}
if (!StringUtils.isEmpty(createTimeEnd)) {
wrapper.le("create_time", createTimeEnd);
}
// 调用mapper的方法
Page<UserInfo> pages = baseMapper.selectPage(pageParam, wrapper);
pages.getRecords().forEach(this::packageUserInfo);
return pages;
}
//编号变成对应值封装
private UserInfo packageUserInfo(UserInfo userInfo) {
//处理认证状态编码
userInfo.getParam().put("authStatusString", AuthStatusEnum.getStatusNameByStatus(userInfo.getAuthStatus()));
//处理用户状态 0 1
userInfo.getParam().put("statusString", userInfo.getStatus() == 0 ? "锁定" : "正常");
return userInfo;
}
...
}
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
# 添加controller方法
创建 com.stt.yygh.user.controller.UserController
类,并调用service方法
package com.stt.yygh.user.controller;
import com.baomidou.mybatisplus.extension.plugins.pagination.Page;
import com.stt.yygh.common.result.Result;
import com.stt.yygh.user.service.UserInfoService;
import com.stt.yygh.vo.user.UserInfoQueryVo;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
@RequestMapping("/admin/user")
public class UserController {
@Autowired
private UserInfoService service;
//用户列表(条件查询带分页)
@GetMapping("{page}/{limit}")
public Result list(@PathVariable Long page,
@PathVariable Long limit,
UserInfoQueryVo userInfoQueryVo) {
return Result.ok(service.selectPage(new Page<>(page, limit), userInfoQueryVo));
}
}
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
# 用户列表 yygh-admin
# 封装api请求
创建 /api/userInfo.js
,添加调用方法
import request from '@/utils/request'
const api_name = '/admin/user'
export function getPageList(page, limit, searchObj) {
return request({
url: `${api_name}/${page}/${limit}`,
method: 'get',
params: searchObj
})
}
2
3
4
5
6
7
8
9
10
11
# 创建用户列表页面
创建/views/user/userInfo/list.vue
组件
<template>
<div class="app-container">
<!--查询表单-->
<el-form :inline="true" class="demo-form-inline">
<el-form-item>
<el-input v-model="searchObj.keyword" placeholder="姓名/手机"/>
</el-form-item>
<el-form-item label="创建时间">
<el-date-picker v-model="searchObj.createTimeBegin" type="datetime"
placeholder="选择开始时间" value-format="yyyy-MM-dd HH:mm:ss" default-time="00:00:00"/>
</el-form-item>
至
<el-form-item>
<el-date-picker v-model="searchObj.createTimeEnd" type="datetime"
placeholder="选择截止时间" value-format="yyyy-MM-dd HH:mm:ss" default-time="00:00:00"/>
</el-form-item>
<el-button type="primary" icon="el-icon-search" @click="fetchData()">查询</el-button>
<el-button type="default" @click="resetData()">清空</el-button>
</el-form>
<!-- 列表 -->
<el-table v-loading="listLoading" :data="list" stripe style="width: 100%">
<el-table-column label="序号" width="70" align="center">
<template slot-scope="scope">{{ (page - 1) * limit + scope.$index + 1 }}</template>
</el-table-column>
<el-table-column prop="phone" label="手机号"/>
<el-table-column prop="nickName" label="昵称"/>
<el-table-column prop="name" label="姓名"/>
<el-table-column label="状态" prop="param.statusString"/>
<el-table-column label="认证状态" prop="param.authStatusString"/>
<el-table-column prop="createTime" label="创建时间"/>
<el-table-column label="操作" width="200" align="center"/>
</el-table>
<!-- 分页组件 -->
<el-pagination :current-page="page" :total="total" :page-size="limit"
:page-sizes="[5, 10, 20, 30, 40, 50, 100]"
style="padding: 30px 0; text-align: center;"
layout="sizes, prev, pager, next, jumper, ->, total, slot"
@current-change="fetchData" @size-change="changeSize"/>
</div>
</template>
<script>
import { getPageList } from '@/api/userInfo'
export default {
// 定义数据
data() {
return {
listLoading: true, // 数据是否正在加载
list: null, // banner列表
total: 0, // 数据库中的总记录数
page: 1, // 默认页码
limit: 10, // 每页记录数
searchObj: {} // 查询表单对象
}
},
// 当页面加载时获取数据
created() {
this.fetchData()
},
methods: {
// 调用api层获取数据库中的数据
fetchData(page = 1) {
this.page = page
this.listLoading = true
getPageList(this.page, this.limit, this.searchObj).then(res => {
this.list = res.data.records
this.total = res.data.total
this.listLoading = false
})
},
// 当页码发生改变的时候
changeSize(size) {
console.log(size)
this.limit = size
this.fetchData(1)
},
// 重置查询表单
resetData() {
console.log('重置查询表单')
this.searchObj = {}
this.fetchData()
}
}
}
</script>
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
# 添加路由
在 src/router/index.js
文件添加路由
{
path: '/user',
component: Layout,
redirect: '/user/userInfo/list',
name: 'userInfo',
meta: { title: '用户管理', icon: 'table' },
alwaysShow: true,
children: [
{
path: 'userInfo/list',
name: '用户列表',
component: () => import('@/views/user/userInfo/list'),
meta: { title: '用户列表', icon: 'table' }
}
]
},
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
# 用户锁定 service-user
# 添加service接口与实现
在com.stt.yygh.user.service.UserInfoService
中添加接口
package com.stt.yygh.user.service;
...
public interface UserInfoService extends IService<UserInfo> {
...
/**
* 用户锁定
* @param userId
* @param status 0:锁定 1:正常
*/
void lock(Long userId, Integer status);
}
2
3
4
5
6
7
8
9
10
11
在UserInfoServiceImpl
类添加实现
package com.stt.yygh.user.service.impl;
...
@Service
public class UserInfoServiceImpl extends ServiceImpl<UserInfoMapper, UserInfo> implements UserInfoService {
...
@Override
public void lock(Long userId, Integer status) {
if(status == 0 || status == 1) {
UserInfo userInfo = this.getById(userId);
userInfo.setStatus(status);
this.updateById(userInfo);
}
}
}
2
3
4
5
6
7
8
9
10
11
12
13
14
# 添加controller方法
在com.stt.yygh.user.controller.UserController
类添加方法
package com.stt.yygh.user.controller;
import com.baomidou.mybatisplus.extension.plugins.pagination.Page;
import com.stt.yygh.common.result.Result;
import com.stt.yygh.user.service.UserInfoService;
import com.stt.yygh.vo.user.UserInfoQueryVo;
import io.swagger.annotations.ApiOperation;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
@RequestMapping("/admin/user")
public class UserController {
@Autowired
private UserInfoService service;
...
@ApiOperation(value = "锁定")
@GetMapping("lock/{userId}/{status}")
public Result lock(@PathVariable("userId") Long userId,
@PathVariable("status") Integer status) {
service.lock(userId, status);
return Result.ok();
}
}
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
# 用户锁定 yygh-admin
# 封装api请求
在 /api/userInfo.js
中,添加调用方法
import request from '@/utils/request'
const api_name = '/admin/user'
...
export function lock(id, status) {
return request({
url: `${api_name}/lock/${id}/${status}`,
method: 'get'
})
}
2
3
4
5
6
7
8
9
10
# 修改页面组件
修改/views/user/userInfo/list.vue
组件,添加操作按键和调用方法
<template>
<div class="app-container">
...
<!-- 列表 -->
<el-table v-loading="listLoading" :data="list" stripe style="width: 100%">
...
<el-table-column label="操作" width="200" align="center">
<template slot-scope="scope">
<el-button v-if="scope.row.status == 1" type="primary" size="mini" @click="lock(scope.row.id, 0)">锁定
</el-button>
<el-button v-if="scope.row.status == 0" type="danger" size="mini" @click="lock(scope.row.id, 1)">取消锁定
</el-button>
</template>
</el-table-column>
</el-table>
<!-- 分页组件 -->
...
</div>
</template>
<script>
import { getPageList, lock } from '@/api/userInfo'
export default {
...
methods: {
...
// 锁定
lock(id, status) {
this.$confirm('确定该操作吗?', '提示', {
confirmButtonText: '确定',
cancelButtonText: '取消',
type: 'warning'
}).then(() => {
return lock(id, status)
}).then((res) => {
this.fetchData(this.page)
if (res.code) {
this.$message({
type: 'success',
message: '操作成功!'
})
}
})
}
}
}
</script>
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
# 用户详情 service-user
详情展示用户信息、用户就诊人信息和登录日志信息
# 添加service接口与实现
在com.stt.yygh.user.service.UserInfoService
中添加接口
package com.stt.yygh.user.service;
...
import java.util.Map;
public interface UserInfoService extends IService<UserInfo> {
...
/**
* 详情
* @param userId
* @return
*/
Map<String, Object> show(Long userId);
}
2
3
4
5
6
7
8
9
10
11
12
13
14
在UserInfoServiceImpl
类添加实现
package com.stt.yygh.user.service.impl;
...
@Service
public class UserInfoServiceImpl extends ServiceImpl<UserInfoMapper, UserInfo> implements UserInfoService {
@Autowired
private RedisTemplate<String, String> redisTemplate;
@Autowired
private PatientService patientService;
...
//用户详情
@Override
public Map<String, Object> show(Long userId) {
Map<String, Object> map = new HashMap<>();
//根据userid查询用户信息
map.put("userInfo", this.packageUserInfo(baseMapper.selectById(userId)));
//根据userid查询就诊人信息
map.put("patientList", patientService.findAllUserId(userId));
return map;
}
}
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
# 添加controller方法
在com.stt.yygh.user.controller.UserController
类添加方法
package com.stt.yygh.user.controller;
...
@RestController
@RequestMapping("/admin/user")
public class UserController {
@Autowired
private UserInfoService service;
...
//用户详情
@GetMapping("show/{userId}")
public Result show(@PathVariable Long userId) {
Map<String,Object> map = service.show(userId);
return Result.ok(map);
}
}
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
# 用户详情 yygh-admin
# 封装api请求
在 /api/userInfo.js
中,添加调用方法
import request from '@/utils/request'
const api_name = '/admin/user'
...
// 用户详情
export function show(id) {
return request({
url: `${api_name}/show/${id}`,
method: 'get'
})
}
2
3
4
5
6
7
8
9
10
11
# 创建用户详情页面
创建/views/user/userInfo/show.vue
组件
<template>
<div class="app-container">
<h4>用户信息</h4>
<table class="table table-striped table-condenseda table-bordered" width="100%">
<tbody>
<tr>
<th width="15%">手机号</th>
<td width="35%"><b>{{ userInfo.phone }}</b></td>
<th width="15%">用户姓名</th>
<td width="35%">{{ userInfo.name }}</td>
</tr>
<tr>
<th>状态</th>
<td>{{ userInfo.status === 0 ? '锁定' : '正常' }}</td>
<th>注册时间</th>
<td>{{ userInfo.createTime }}</td>
</tr>
</tbody>
</table>
<h4>认证信息</h4>
<table class="table table-striped table-condenseda table-bordered" width="100%">
<tbody>
<tr>
<th width="15%">姓名</th>
<td width="35%"><b>{{ userInfo.name }}</b></td>
<th width="15%">证件类型</th>
<td width="35%">{{ userInfo.certificatesType }}</td>
</tr>
<tr>
<th>证件号</th>
<td>{{ userInfo.certificatesNo }}</td>
<th>证件图片</th>
<td><img :src="userInfo.certificatesUrl" width="80px"></td>
</tr>
</tbody>
</table>
<h4>就诊人信息</h4>
<el-table v-loading="listLoading" :data="patientList" stripe style="width: 100%">
<el-table-column label="序号" width="70" align="center">
<template slot-scope="scope">{{ scope.$index + 1 }}</template>
</el-table-column>
<el-table-column prop="name" label="姓名"/>
<el-table-column prop="param.certificatesTypeString" label="证件类型"/>
<el-table-column prop="certificatesNo" label="证件编号"/>
<el-table-column label="性别">
<template slot-scope="scope">{{ scope.row.sex == 1 ? '男' : '女' }}</template>
</el-table-column>
<el-table-column prop="birthdate" label="出生年月"/>
<el-table-column prop="phone" label="手机"/>
<el-table-column label="是否结婚">
<template slot-scope="scope">{{ scope.row.isMarry == 1 ? '时' : '否' }}</template>
</el-table-column>
<el-table-column prop="fullAddress" label="地址"/>
<el-table-column prop="createTime" label="注册时间"/>
</el-table>
<el-row>
<el-button @click="back">返回</el-button>
</el-row>
</div>
</template>
<script>
import { show } from '@/api/userInfo'
export default {
// 定义数据
data() {
return {
id: this.$route.params.id,
userInfo: {}, // 会员信息
patientList: [] // 就诊人列表
}
},
created() {
this.fetchDataById()
},
methods: {
fetchDataById() {
show(this.id).then(res => {
this.userInfo = res.data.userInfo
this.patientList = res.data.patientList
})
},
back() {
window.history.back(-1)
}
}
}
</script>
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
# 添加路由
在 src/router/index.js
文件添加路由
{
path: '/user',
component: Layout,
redirect: '/user/userInfo/list',
name: 'userInfo',
meta: { title: '用户管理', icon: 'table' },
alwaysShow: true,
children: [
...
{
path: 'userInfo/show/:id',
name: '用户查看',
component: () => import('@/views/user/userInfo/show'),
meta: { title: '用户查看' },
hidden: true
}
]
},
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
# 修改用户列表添加路由按键
<template>
<div class="app-container">
...
<!-- 列表 -->
<el-table v-loading="listLoading" :data="list" stripe style="width: 100%">
...
<el-table-column label="操作" width="200" align="center">
<template slot-scope="scope">
<router-link :to="'/user/userInfo/show/'+scope.row.id">
<el-button type="primary" size="mini">查看</el-button>
</router-link>
<el-button v-if="scope.row.status === 1" type="primary" size="mini" @click="lock(scope.row.id, 0)">
锁定
</el-button>
<el-button v-if="scope.row.status === 0" type="danger" size="mini" @click="lock(scope.row.id, 1)">
取消锁定
</el-button>
</template>
</el-table-column>
</el-table>
<!-- 分页组件 -->
...
</div>
</template>
<script>
...
</script>
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
# 用户认证列表 yygh-admin
api接口与用户列表一致,只是默认加了一个认证状态搜索条件:authStatus
# 创建用户认证列表页面
创建/views/user/userInfo/authList.vue
组件
<template>
<div class="app-container">
<!--查询表单-->
<el-form :inline="true" class="demo-form-inline">
<el-form-item>
<el-input v-model="searchObj.keyword" placeholder="姓名/手机"/>
</el-form-item>
<el-form-item label="创建时间">
<el-date-picker v-model="searchObj.createTimeBegin" type="datetime" placeholder="选择开始时间"
value-format="yyyy-MM-dd HH:mm:ss" default-time="00:00:00"/>
</el-form-item>
至
<el-form-item>
<el-date-picker v-model="searchObj.createTimeEnd" type="datetime" placeholder="选择截止时间"
value-format="yyyy-MM-dd HH:mm:ss" default-time="00:00:00"/>
</el-form-item>
<el-button type="primary" icon="el-icon-search" @click="fetchData()">查询</el-button>
<el-button type="default" @click="resetData()">清空</el-button>
</el-form>
<!-- 列表 -->
<el-table v-loading="listLoading" :data="list" stripe style="width: 100%">
<el-table-column label="序号" width="70" align="center">
<template slot-scope="scope">{{ (page - 1) * limit + scope.$index + 1 }}</template>
</el-table-column>
<el-table-column prop="name" label="姓名"/>
<el-table-column prop="certificatesType" label="证件类型"/>
<el-table-column prop="certificatesNo" label="证件号"/>
<el-table-column prop="createTime" label="创建时间"/>
<el-table-column label="操作" width="250" align="center">
<template slot-scope="scope">
<router-link :to="'/user/userInfo/show/'+scope.row.id">
<el-button type="primary" size="mini">查看</el-button>
</router-link>
</template>
</el-table-column>
</el-table>
<!-- 分页组件 -->
<el-pagination :current-page="page" :total="total" :page-size="limit" :page-sizes="[5, 10, 20, 30, 40, 50, 100]"
style="padding: 30px 0; text-align: center;"
layout="sizes, prev, pager, next, jumper, ->, total, slot"
@current-change="fetchData"
@size-change="changeSize"/>
</div>
</template>
<script>
import { getPageList } from '@/api/userInfo'
export default {
data() {
return {
listLoading: true, // 数据是否正在加载
list: null, // banner列表
total: 0, // 数据库中的总记录数
page: 1, // 默认页码
limit: 10, // 每页记录数
searchObj: {
authStatus: 1
} // 查询表单对象
}
},
created() {
this.fetchData()
},
methods: {
fetchData(page = 1) {
this.page = page
this.listLoading = true
getPageList(this.page, this.limit, this.searchObj).then(res => {
this.list = res.data.records
this.total = res.data.total
this.listLoading = false
}).catch(() => {
this.listLoading = false
})
},
changeSize(size) {
this.limit = size
this.fetchData(1)
},
resetData() {
console.log('重置查询表单')
this.searchObj = {}
this.fetchData()
}
}
}
</script>
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
# 添加路由
在 src/router/index.js
中添加路由
{
path: '/user',
component: Layout,
redirect: '/user/userInfo/list',
name: 'userInfo',
meta: { title: '用户管理', icon: 'table' },
alwaysShow: true,
children: [
...
{
path: 'userInfo/authList',
name: '认证审批列表',
component: () => import('@/views/user/userInfo/authList'),
meta: { title: '认证审批列表', icon: 'table' }
}
]
},
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
# 用户认证审批 service-user
# 添加service接口与实现
在com.stt.yygh.user.service.UserInfoService
中添加接口
package com.stt.yygh.user.service;
...
public interface UserInfoService extends IService<UserInfo> {
...
/**
* 认证审批
* @param userId
* @param authStatus 2:通过 -1:不通过
*/
void approve(Long userId, Integer authStatus);
}
2
3
4
5
6
7
8
9
10
11
12
在UserInfoServiceImpl
类添加实现
package com.stt.yygh.user.service.impl;
...
@Service
public class UserInfoServiceImpl extends ServiceImpl<UserInfoMapper, UserInfo> implements UserInfoService {
...
// 认证审批 2通过 -1不通过
@Override
public void approve(Long userId, Integer authStatus) {
if(authStatus == 2 || authStatus == -1) {
UserInfo userInfo = baseMapper.selectById(userId);
userInfo.setAuthStatus(authStatus);
baseMapper.updateById(userInfo);
}
}
}
2
3
4
5
6
7
8
9
10
11
12
13
14
15
# 添加controller方法
在com.stt.yygh.user.controller.UserController
类添加方法
package com.stt.yygh.user.controller;
...
@RestController
@RequestMapping("/admin/user")
public class UserController {
@Autowired
private UserInfoService service;
...
//认证审批
@GetMapping("approve/{userId}/{authStatus}")
public Result approval(@PathVariable Long userId,@PathVariable Integer authStatus) {
service.approve(userId,authStatus);
return Result.ok();
}
}
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
# 用户认证审批 yygh-admin
# 封装api请求
在 /api/userInfo.js
中,添加调用方法
import request from '@/utils/request'
const api_name = '/admin/user'
...
// 认证审批
export function approve(id, authStatus) {
return request({
url: `${api_name}/approve/${id}/${authStatus}`,
method: 'get'
})
}
2
3
4
5
6
7
8
9
10
11
12
# 修改页面
修改/views/user/userInfo/authList.vue
组件,添加操作按键和调用方法
<template>
<div class="app-container">
...
<!-- 列表 -->
<el-table v-loading="listLoading" :data="list" stripe style="width: 100%">
...
<el-table-column label="操作" width="250" align="center">
<template slot-scope="scope">
<router-link :to="'/user/userInfo/show/'+scope.row.id">
<el-button type="primary" size="mini">查看</el-button>
</router-link>
<el-button type="primary" size="mini" @click="approve(scope.row.id, 2)">通过</el-button>
<el-button type="danger" size="mini" @click="approve(scope.row.id, -1)">不通过</el-button>
</template>
</el-table-column>
</el-table>
<!-- 分页组件 -->
...
</div>
</template>
<script>
import { approve, getPageList } from '@/api/userInfo'
export default {
...
methods: {
...
approve(id, authStatus) {
this.$confirm('确定该操作吗?', '提示', {
confirmButtonText: '确定',
cancelButtonText: '取消',
type: 'warning'
}).then(() => {
return approve(id, authStatus)
}).then((res) => {
this.fetchData(this.page)
if (res.code) {
this.$message({
type: 'success',
message: '操作成功!'
})
}
})
}
}
}
</script>
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51