33.后台系统-订单管理
# 需求分析
之前实现了预约下单功能,创建完成订单后,要在管理员可以进行订单的管理
# 订单列表
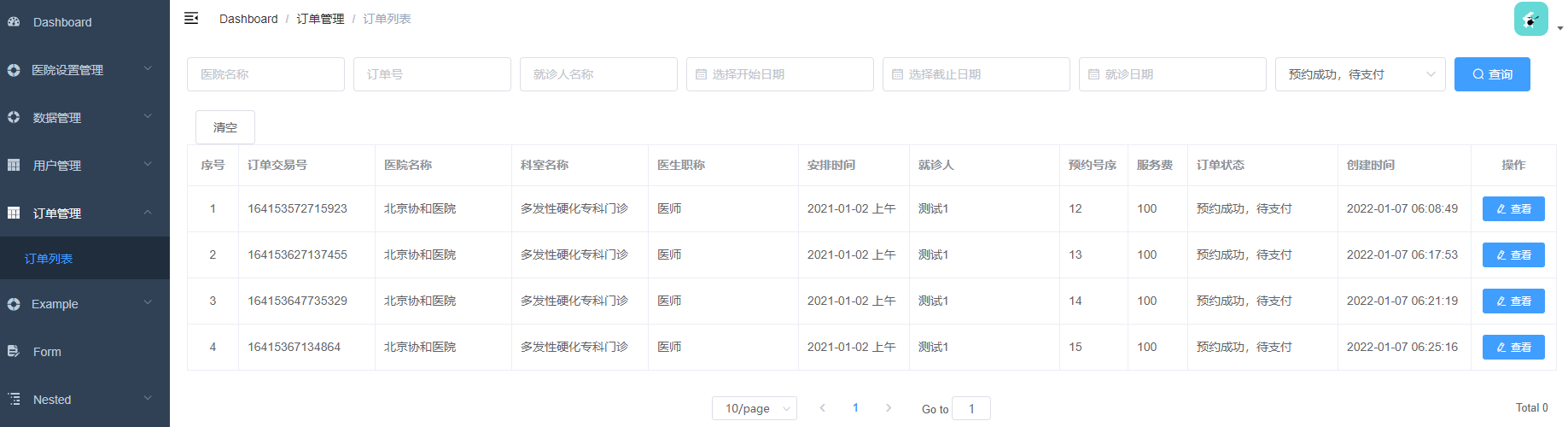
# 订单详情
点击查看触发此页面
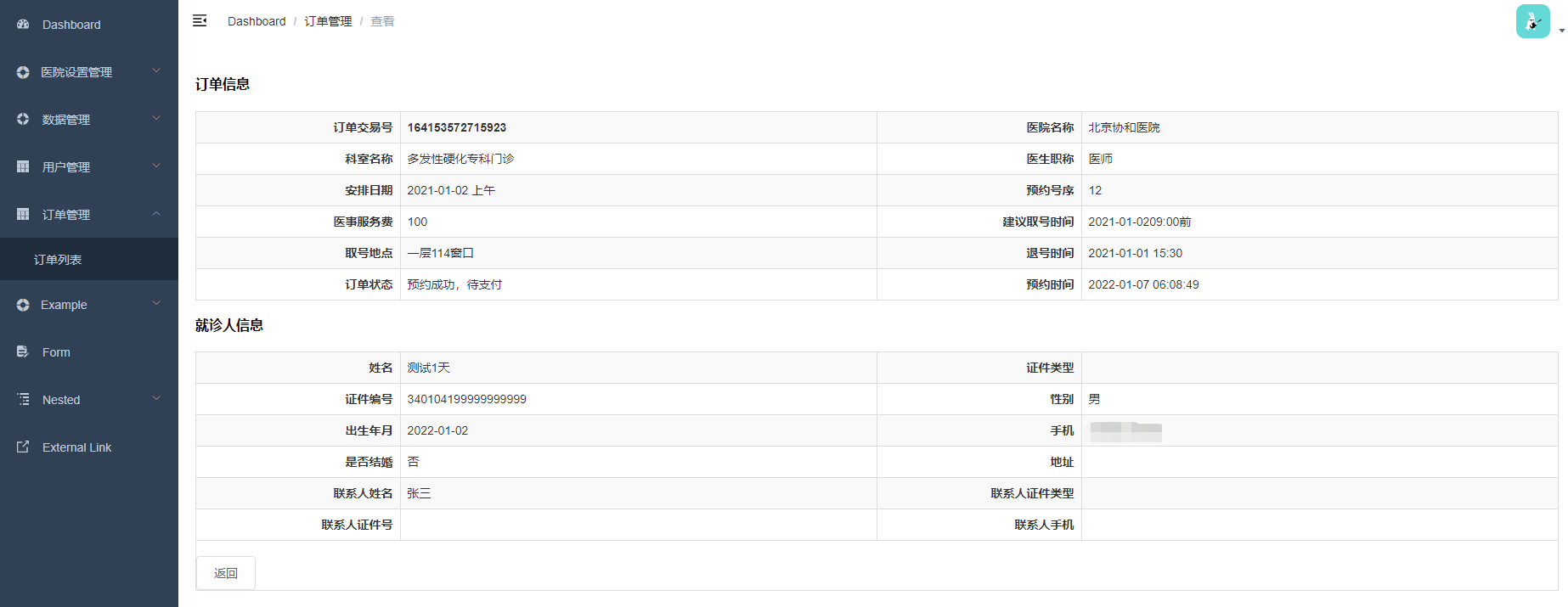
# 订单列表 service-order
添加com.stt.yygh.order.controller.OrderController
类
package com.stt.yygh.order.controller;
import com.baomidou.mybatisplus.extension.plugins.pagination.Page;
import com.stt.yygh.common.result.Result;
import com.stt.yygh.enums.OrderStatusEnum;
import com.stt.yygh.model.order.OrderInfo;
import com.stt.yygh.order.service.OrderService;
import com.stt.yygh.vo.order.OrderQueryVo;
import io.swagger.annotations.Api;
import io.swagger.annotations.ApiOperation;
import io.swagger.annotations.ApiParam;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@Api(tags = "订单接口")
@RestController
@RequestMapping("/admin/order/orderInfo")
public class OrderController {
@Autowired
private OrderService service;
@ApiOperation(value = "获取分页列表")
@GetMapping("{page}/{limit}")
public Result index(@ApiParam(name = "page", value = "当前页码", required = true) @PathVariable Long page,
@ApiParam(name = "limit", value = "每页记录数", required = true) @PathVariable Long limit,
@ApiParam(name = "orderCountQueryVo", value = "查询对象") OrderQueryVo orderQueryVo) {
Page<OrderInfo> pageModel = service.selectPage(new Page<>(page, limit), orderQueryVo);
return Result.ok(pageModel);
}
@ApiOperation(value = "获取订单状态")
@GetMapping("getStatusList")
public Result getStatusList() {
return Result.ok(OrderStatusEnum.getStatusList());
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
# 订单列表 yygh-admin
# 添加路由
在 src/router/index.js 文件添加路由
{
path: '/order',
component: Layout,
redirect: '/order/orderInfo/list',
name: 'BasesInfo',
meta: { title: '订单管理', icon: 'table' },
alwaysShow: true,
children: [
{
path: 'orderInfo/list',
name: '订单列表',
component: () => import('@/views/order/orderInfo/list'),
meta: { title: '订单列表' }
}
]
},
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
# 封装api
添加/api/orderInfo.js
文件
import request from '@/utils/request'
const api_name = '/admin/order/orderInfo'
export default {
getPageList(page, limit, searchObj) {
return request({
url: `${api_name}/${page}/${limit}`,
method: 'get',
params: searchObj
})
},
getStatusList() {
return request({
url: `${api_name}/getStatusList`,
method: 'get'
})
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
# 添加页面组件
创建/views/order/orderInfo/list.vue组件
- 注意:查看页面已经添加了跳转到详情的路由
<template>
<div class="app-container">
<el-form :inline="true" class="demo-form-inline">
<el-form-item>
<el-input v-model="searchObj.hosname" placeholder="医院名称"/>
</el-form-item>
<el-form-item>
<el-input v-model="searchObj.outTradeNo" placeholder="订单号"/>
</el-form-item>
<el-form-item>
<el-input v-model="searchObj.patientName" placeholder="就诊人名称"/>
</el-form-item>
<el-form-item>
<el-date-picker v-model="searchObj.createTimeBegin" type="date" placeholder="选择开始日期" value-format="yyyy-MM-dd"/>
</el-form-item>
<el-form-item>
<el-date-picker v-model="searchObj.createTimeEnd" type="date" placeholder="选择截止日期" value-format="yyyy-MM-dd"/>
</el-form-item>
<el-form-item>
<el-date-picker v-model="searchObj.reserveDate" type="date" placeholder="就诊日期" value-format="yyyy-MM-dd"/>
</el-form-item>
<el-form-item>
<el-select v-model="searchObj.orderStatus" placeholder="订单状态" class="v-select patient-select">
<el-option v-for="item in statusList" :key="item.status" :label="item.comment" :value="item.status"/>
</el-select>
</el-form-item>
<el-button type="primary" icon="el-icon-search" @click="fetchData()">查询</el-button>
<el-button type="default" @click="resetData()">清空</el-button>
</el-form>
<!-- 列表 -->
<el-table v-loading="listLoading" :data="list" border fit highlight-current-row>
<el-table-column label="序号" width="60" align="center">
<template slot-scope="scope">{{ (page - 1) * limit + scope.$index + 1 }}</template>
</el-table-column>
<el-table-column prop="outTradeNo" label="订单交易号" width="160"/>
<el-table-column prop="hosname" label="医院名称" width="160"/>
<el-table-column prop="depname" label="科室名称" width="160"/>
<el-table-column prop="title" label="医生职称"/>
<el-table-column label="安排时间" width="130">
<template slot-scope="scope">
{{ scope.row.reserveDate }} {{ scope.row.reserveTime === 0 ? '上午' : '下午' }}
</template>
</el-table-column>
<el-table-column prop="patientName" label="就诊人"/>
<el-table-column prop="number" label="预约号序" width="80"/>
<el-table-column prop="amount" label="服务费" width="70"/>
<el-table-column prop="param.orderStatusString" label="订单状态"/>
<el-table-column prop="createTime" label="创建时间" width="156"/>
<el-table-column label="操作" width="100" align="center">
<template slot-scope="scope">
<router-link :to="'/order/orderInfo/show/'+scope.row.id">
<el-button type="primary" size="mini" icon="el-icon-edit">查看</el-button>
</router-link>
</template>
</el-table-column>
</el-table>
<!-- 分页组件 -->
<el-pagination :current-page="page" :total="total" :page-size="limit" :page-sizes="[5, 10, 20, 30, 40, 50, 100]"
style="padding: 30px 0; text-align: center;"
layout="sizes, prev, pager, next, jumper, ->, total, slot"
@current-change="fetchData" @size-change="changeSize"/>
</div>
</template>
<script>
import orderInfoApi from '@/api/orderInfo'
export default {
data() {
return {
listLoading: true, // 数据是否正在加载
list: null, // banner列表
total: 0, // 数据库中的总记录数
page: 1, // 默认页码
limit: 10, // 每页记录数
searchObj: {} // 查询表单对象
}
},
// 生命周期函数:内存准备完毕,页面尚未渲染
created() {
this.fetchData()
this.getStatusList()
},
methods: {
// 当页码发生改变的时候
changeSize(size) {
this.limit = size
this.fetchData(1)
},
// 加载banner列表数据
fetchData(page = 1) {
this.page = page
this.listLoading = true
orderInfoApi.getPageList(this.page, this.limit, this.searchObj).then(res => {
this.list = res.data.records
this.total = res.data.total
// 数据加载并绑定成功
this.listLoading = false
})
},
getStatusList() {
orderInfoApi.getStatusList().then(res => {
this.statusList = res.data
})
},
// 重置查询表单
resetData() {
this.searchObj = {}
this.fetchData()
}
}
}
</script>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
# 订单详情 service-order
# 添加service方法
在OrderService
中添加接口
package com.stt.yygh.order.service;
...
public interface OrderService extends IService<OrderInfo> {
...
// 订单详情 给后台系统使用
Map<String, Object> show(Long orderId);
}
1
2
3
4
5
6
7
2
3
4
5
6
7
对应的实现
package com.stt.yygh.order.service.impl;
import com.alibaba.fastjson.JSONObject;
import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper;
import com.baomidou.mybatisplus.extension.plugins.pagination.Page;
import com.baomidou.mybatisplus.extension.service.impl.ServiceImpl;
import com.stt.yygh.common.exception.YyghException;
import com.stt.yygh.common.helper.HttpRequestHelper;
import com.stt.yygh.common.result.ResultCodeEnum;
import com.stt.yygh.enums.OrderStatusEnum;
import com.stt.yygh.hosp.client.HospitalFeignClient;
import com.stt.yygh.model.order.OrderInfo;
import com.stt.yygh.model.user.Patient;
import com.stt.yygh.order.mapper.OrderInfoMapper;
import com.stt.yygh.order.service.OrderService;
import com.stt.yygh.rabbitmq.MqConst;
import com.stt.yygh.rabbitmq.RabbitService;
import com.stt.yygh.user.client.PatientFeignClient;
import com.stt.yygh.vo.order.OrderMqVo;
import com.stt.yygh.vo.hosp.ScheduleOrderVo;
import com.stt.yygh.vo.hosp.SignInfoVo;
import com.stt.yygh.vo.msm.MsmVo;
import com.stt.yygh.vo.order.OrderQueryVo;
import org.joda.time.DateTime;
import org.springframework.beans.BeanUtils;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import org.springframework.transaction.annotation.Transactional;
import org.springframework.util.StringUtils;
import java.util.HashMap;
import java.util.Map;
import java.util.Objects;
import java.util.Random;
@Service
public class OrderServiceImpl extends ServiceImpl<OrderInfoMapper, OrderInfo> implements OrderService {
@Autowired
private PatientFeignClient patientFeignClient;
...
@Override
public Map<String, Object> show(Long orderId) {
Map<String, Object> map = new HashMap<>();
OrderInfo orderInfo = this.packOrderInfo(this.getById(orderId));
map.put("orderInfo", orderInfo);
Patient patient = patientFeignClient.getPatientInfo(orderInfo.getPatientId());
map.put("patient", patient);
return map;
}
...
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
# 添加controller方法
在OrderController
类添加方法
package com.stt.yygh.order.controller;
...
@Api(tags = "订单接口")
@RestController
@RequestMapping("/admin/order/orderInfo")
public class OrderController {
@Autowired
private OrderService service;
...
@ApiOperation(value = "获取订单")
@GetMapping("show/{id}")
public Result get(@ApiParam(name = "orderId", value = "订单id", required = true) @PathVariable Long id) {
return Result.ok(service.show(id));
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
2
3
4
5
6
7
8
9
10
11
12
13
14
15
# 订单详情 yygh-admin
# 添加路由
在 src/router/index.js 文件添加路由
{
path: '/order',
component: Layout,
redirect: '/order/orderInfo/list',
name: 'BasesInfo',
meta: { title: '订单管理', icon: 'table' },
alwaysShow: true,
children: [
{
path: 'orderInfo/list',
name: '订单列表',
component: () => import('@/views/order/orderInfo/list'),
meta: { title: '订单列表' }
},
{
path: 'orderInfo/show/:id',
name: '查看',
component: () => import('@/views/order/orderInfo/show'),
meta: { title: '查看', noCache: true },
hidden: true
}
]
},
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
# 封装api
在/api/orderInfo.js
文件添加方法
import request from '@/utils/request'
const api_name = '/admin/order/orderInfo'
export default {
..,
getById(id) {
return request({
url: `${api_name}/show/${id}`,
method: 'get'
})
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
2
3
4
5
6
7
8
9
10
11
12
13
# 添加页面组件
创建/views/order/orderInfo/show.vue组件
<template>
<div class="app-container">
<h4>订单信息</h4>
<table class="table table-striped table-condenseda table-bordered" width="100%">
<tbody>
<tr>
<th width="15%">订单交易号</th>
<td width="35%"><b style="font-size: 14px">{{ orderInfo.outTradeNo }}</b></td>
<th width="15%">医院名称</th>
<td width="35%">{{ orderInfo.hosname }}</td>
</tr>
<tr>
<th>科室名称</th>
<td>{{ orderInfo.depname }}</td>
<th>医生职称</th>
<td>{{ orderInfo.title }}</td>
</tr>
<tr>
<th>安排日期</th>
<td>{{ orderInfo.reserveDate }} {{ orderInfo.reserveTime === 0 ? '上午' : '下午' }}</td>
<th>预约号序</th>
<td>{{ orderInfo.number }}</td>
</tr>
<tr>
<th>医事服务费</th>
<td>{{ orderInfo.amount }}</td>
<th>建议取号时间</th>
<td>{{ orderInfo.fetchTime }}</td>
</tr>
<tr>
<th>取号地点</th>
<td>{{ orderInfo.fetchAddress }}</td>
<th>退号时间</th>
<td>{{ orderInfo.quitTime }}</td>
</tr>
<tr>
<th>订单状态</th>
<td>{{ orderInfo.param.orderStatusString }}</td>
<th>预约时间</th>
<td>{{ orderInfo.createTime }}</td>
</tr>
</tbody>
</table>
<h4>就诊人信息</h4>
<table class="table table-striped table-condenseda table-bordered" width="100%">
<tbody>
<tr>
<th width="15%">姓名</th>
<td width="35%">{{ patient.name }}天</td>
<th width="15%">证件类型</th>
<td width="35%">{{ patient.param.certificatesTypeString }}</td>
</tr>
<tr>
<th>证件编号</th>
<td>{{ patient.certificatesNo }}</td>
<th>性别</th>
<td>{{ patient.sex === 1 ? '男' : '女' }}</td>
</tr>
<tr>
<th>出生年月</th>
<td>{{ patient.birthdate }}</td>
<th>手机</th>
<td>{{ patient.phone }}</td>
</tr>
<tr>
<th>是否结婚</th>
<td>{{ patient.isMarry === 1 ? '是' : '否' }}</td>
<th>地址</th>
<td>{{ patient.param.fullAddress }}</td>
</tr>
<tr>
<th>联系人姓名</th>
<td>{{ patient.contactsName }}</td>
<th>联系人证件类型</th>
<td>{{ patient.param.contactsCertificatesTypeString }}</td>
</tr>
<tr>
<th>联系人证件号</th>
<td>{{ orderInfo.contactsCertificatesNo }}</td>
<th>联系人手机</th>
<td>{{ orderInfo.contactsPhone }}</td>
</tr>
<br>
<el-row>
<el-button @click="back">返回</el-button>
</el-row>
</tbody>
</table>
</div>
</template>
<script>
// 引入组件
import orderInfoApi from '@/api/orderInfo'
export default {
data() {
return {
orderInfo: null,
patient: null
}
},
// 生命周期方法(在路由切换,组件不变的情况下不会被调用)
created() {
this.init()
},
methods: {
// 表单初始化
init() {
const id = this.$route.params.id
this.fetchDataById(id)
},
// 根据id查询记录
fetchDataById(id) {
orderInfoApi.getById(id).then(res => {
this.orderInfo = res.data.orderInfo
this.patient = res.data.patient
})
},
back() {
this.$router.push({ path: '/order/orderInfo/list' })
}
}
}
</script>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
Last Updated: 2022/01/16, 11:29:51